Welcome to part 9 of this blog series introducing
abap2UI5 — an open-source project for developing UI5 apps purely in ABAP.
In the last few months, a bunch of new features – both big and small – have been added to abap2UI5. Some were in response to issues, some from pull requests and some were inspired by SAP UI5 documentation. These all work to further enhance the functionality of abap2UI5 – which we'll explore in this blog post.
We'll begin with tree controls and move on to partial rerendering with model updates & nested views. Then we explore the use of dynamically created data models, for example, for importing CSVs. Additionally, we'll examine additional UI5 controls like Navigation Container, Gantt Charts, Planning Calendars, Message Managers, and extensions related to Excel Downloads and Generic Search Helps. Finally, we'll delve into a full demo app and explore the use of abap2UI5 in different ABAP extensibility scenarios.
Blog Series & More
You can find all the information about this project on
GitHub and stay up-to-date by following on
Twitter. Also, make sure to explore the other articles in this blog series.
Content
This post covers the following areas:
- Tree Controls
- Partly View Rendering with Model Updates
- Partly View Rendering with Nested Views
- Displaying Dynamic Data with S-RTTI
- CSV Upload/Download
- Excel Upload/Download
- Navigation Container
- Visualization with Planning Calender, Gantt Charts and Process Flows
- Message Manager
- Generic Search Help
- Developer Code Challenge
- Demo & Samples Repository
- Conclusion
Let’s begin with the first topic.
1. Tree Controls
In addition to displaying tables and lists, it is now also possible to use controls based on tree models. This enables the visualization of hierarchies:

abap2UI5 - Displaying UI5 Tree Controls
Among many other use cases, tree structures can also be used to create popups for selecting items:
abap2UI5 - Popup with Tree Control to Select an Item
To generate the output shown above, you need to define a nested structure in ABAP as follows:
TYPES:
BEGIN OF ts_tree_row_base,
object TYPE string,
col2 TYPE string,
col3 TYPE string,
col4 TYPE string,
END OF ts_tree_row_base.
TYPES:
BEGIN OF ts_tree_level2.
INCLUDE TYPE ts_tree_row_base,
END OF ts_tree_level2.
TYPES tt_tree_level2 TYPE STANDARD TABLE OF ts_tree_level2 WITH KEY object.
TYPES:
BEGIN OF ts_tree_level1,
INCLUDE TYPE ts_tree_row_base,
categories TYPE tt_tree_level2.
END OF ts_tree_level1.
TYPES tt_tree_level1 TYPE STANDARD TABLE OF ts_tree_level1 WITH KEY object.
And the
UI5 Tree Control is used in the view definition:
DATA(tab) = page->tree_table(
rows = `{path:'` && client->_bind( val = mt_tree path = abap_true ) &&
`', parameters: {arrayNames:['CATEGORIES']}}` ).
tab->tree_columns( )->tree_column( label = 'Object'
)->tree_template(
)->text( text = '{OBJECT}')->get_parent( )->get_parent(
)->tree_column( label = 'Column2'
)->tree_template(
)->text( text = '{COL2}')->get_parent( )->get_parent(
)->tree_column( label = 'Column3'
)->tree_template(
)->text( text = '{COL3}')->get_parent( )->get_parent(
)->tree_column( label = 'Column4'
)->tree_template(
)->text( text = '{COL4}').
You can check the full source code of these examples
here and
here. Thank you to
axelmohnen and
WegnerDan for their contributions and examples in this direction.
2. Partly View Rendering I (Model Update)
In the initial version of abap2UI5, the standard approach involved rerendering the entire view after each request. This approach can be beneficial when making full changes to the entire application or when the entire UI changes with a page transition. However, in cases where only specific entries in a table or the values of an input field need to change, repeatedly rerendering the entire view can result in an uncomfortable user experience. For example it disrupts the user's current focus and scroll position. Therefore, abap2UI5 has now been extended to allow updating only the view model and keeping the view stable during a backend request. Rerendering is now controlled by two different methods:
- view_display - This method rerenders the entire view
- view_model_update - This method updates only the view model, and the UI5 framework will rerender only the affected controls
Let's consider the following example: Initially, a new view is created with a table and a search field. Subsequently, with each input change, the backend sends the newly matching table, and only the table entries in the UI are rerendered. The rest of the UI remains stable and the focus also stays unchanged in the input field:
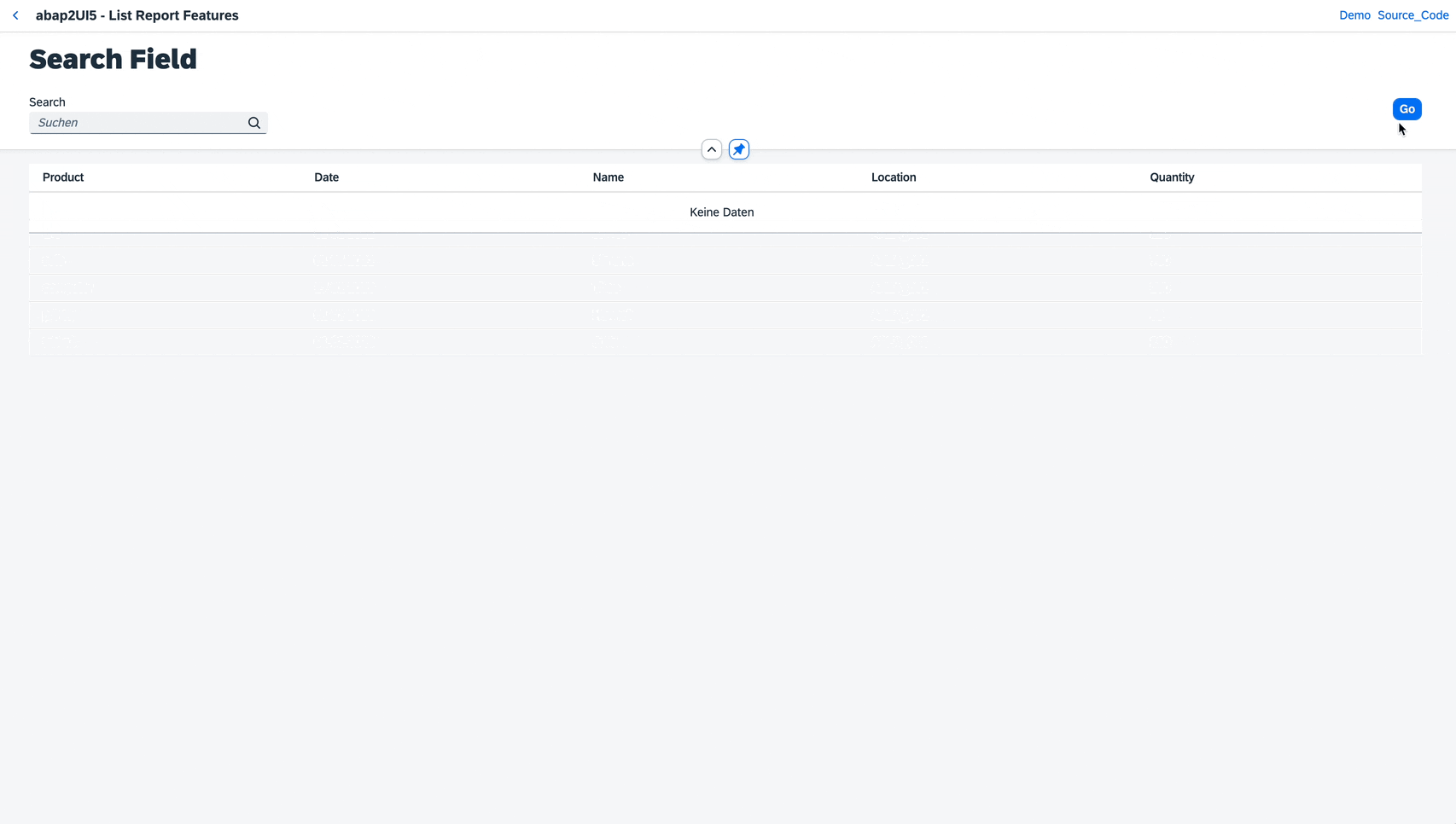
abap2UI5 - Model Update after Input Change
Check out the source code
here. This becomes even more useful when you have a table and have scrolled to a certain position. Take a look at
this demo where new entries are added to a table every few seconds, and the scroll position and focus remain unchanged:

abap2UI5 - Stable Focus and Scroll Position after Backend Model Updates
As a general recommendation, it is advisable not to rerender the whole view unnecessarily, but only when it is required. While some UI5 Controls may need a complete rerendering, most cases can avoid it. However, it is essential to ensure that the entire view is rerendered after leaving or returning to an app. For this purpose, the event 'on_navigated' can now be checked, which is always true after an app navigation:
if client->get( )-check_on_navigated = abap_true.
"rerender the whole View
endif.
Next, let's explore another method for partial rerendering.
3. Partly View Rendering II (Nested Views)
Another approach for selectively rerendering views involves using nested views. This allows you to create, for example, a master-detail page. You can use two independent views displaying a tree control on the left side and another view with inputs and buttons on the right side. Take a look at
this example:

abap2UI5 - Master-Detail Layout with Nested Views
Many other use cases are possible. To mention just one more, you can display two tables and move entries from the left one to the right table:
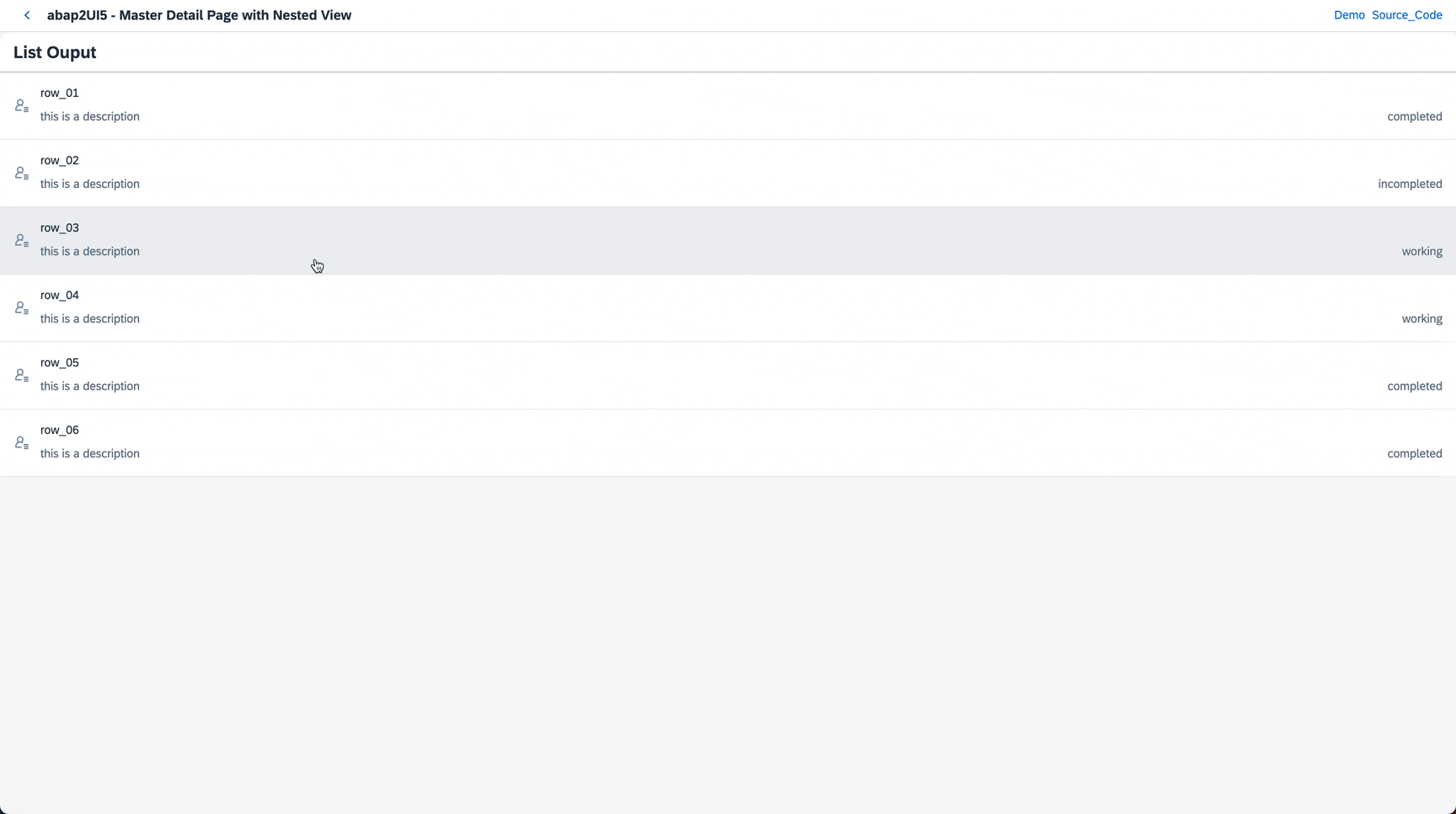
abap2UI5 - Nested Views with Interacting Tables
Check out the source code
here. The nested view is added to the main view with the following function:
client->nest_view_display(
val = lo_view_nested->stringify( )
id = `ParentView`
method_insert = 'addMidColumnPage'
method_destroy = 'removeAllMidColumnPages'
).
And the methods to rerender the nested view are similar to the ones of the main view:
- nest_view_display - This method rerenders the entire nested view
- nest_view_model_update - This method updates only the view model, and the UI5 framework will rerender only the affected controls in the nested view
A big thank you to
choper725 for the example and testing abap2UI5 with nested views.
4. Displaying Generic Data References with S-RTTI
One common requirement in ABAP is displaying tables which are typed during runtime. Sometimes you only know during runtime which database table you need to display or have to create a table with RTTI depending on local created types. When it comes to ALVs, this is not a problem because you can also create field catalogs dynamically. Here the abap2UI5 approach reaches its limits because we serialize the app with XSLT after every request and unfortunately generic data references with locally created types cause XSLT errors during serialization:

XSLT Serialization Error - Locally Created Types not supported
Fortunately the open source project
S-RTTI solves this problem:

Open Source Project S-RTTI
With S-RTTI, we can serialize and save the RTTI of every variable, enabling us to clear it at the end of each request. Then, with the next request, the variable is recreated again based on the S-RTTI information. When encountering XSLT errors, abap2UI5 now automatically checks if this framework is installed and uses S-RTTI for serialization. As a result, generic data references with locally created types can now be seamlessly used with abap2UI5. Many thanks to
sandra.rossi for this idea and excellent
project.
5. CSV Upload & Download
One compelling use case for the functionality described above is the import of tables via CSV. The following application reads the file and, based on its contents, creates an internal table with the data from the CSV. Finally, it displays the table in a UI5 Table Control. Take a look at this example:

abap2UI5 - Upload CSV and Create Internal Table with its Content
By utilizing S-RTTI in combination with abap2UI5, only about 153 lines of ABAP are needed to fulfill this requirement. You can check out the source code
here.
6. Excel Upload & Download
Besides CSV, another common requirement is the use of Excel files, especially downloading internal tables as Excel files. In UI5, this is achieved using the
Spreadsheet Control. However, they rely on frontend JavaScript logic and are not usable without additional work in abap2UI5. Fortunately,
choper725 contributed a custom control that encapsulates the frontend logic within a UI5 control, making it usable in a view definition of abap2UI5. Take a look at this example:

abap2UI5 - Excel Download via Custom Control
You can find the logic of the custom control
here and the source code of the demo
here. This approach combines JavaScript Logic at the frontend with ABAP Logic at the backend. Thank you
choper725 for this extension.
Another approach for handling Excel files could be to keep the entire logic in the backend and send and receive only the final file to the frontend, similar to what we did with the CSV. You can achieve this by using the project
abap2xlsx combined with abap2UI5. Currently, there is no working Excel functionality usable in both language versions ('ABAP for Cloud' and 'Standard ABAP'), but maybe in the future, there will be a chance for this, allowing for the entire logic to be held in ABAP without the need for extra JavaScript or the use of UI5 Spreadsheet Controls (pure ABAP
😍). Feel free to support this idea
here.
Next let's explore some more UI5 Controls.
7. Navigation Container
First we take a look at the
UI5 Navigation Container. Check out this demo:

abap2UI5 - nav container
You can find the source code
here. The Navigation Container allows you to structure your output with multiple views, switching between them using an event. You can achieve this behavior by calling the following method in abap2UI5:
client->_event_client( val = 'NAV_TO' t_arg = VALUE #( ( `NavCon` ) ( mv_selected_key ) ) ).
Furthermore, a lot of new controls for visualization are available.
8. Visualization with Planning Calender, Gantt Chart and Process Flow
Look for example at this demo with
UI5 Gantt Charts:

abap2UI5 - Gantt Chart
Check out the source code
here. Or the
UI5 Planning Calender:

abap2UI5 - Planning Calender
Check out the source code
here. And the
UI5 Process Flow:

abap2UI5 - Process Flow
Check out the source code
here. All of these controls assist in visualizing your data in a format other than a table or list. Thank you
choper725 for these extensions.
9. Message Manager
Message Handling can be done in UI5 with the
Message Manager. Depending an input field types it is automatically filled with message and the output is seamlessly integrated to the UI. Look at this demo:

abap2UI5 - Message Manager
In abap2UI5, you can now add messages, delete messages, or read its messages. Thank you
choper725 for the demo, you can find the source code
here.
10. Generic Search Help
When users input data, in addition to the message manager, search helps are a valuable assistance to fill input fields. While, of course, with abap2UI5, you have the capability to create your own popups and develop search helps manually, wouldn't it be nice to leverage the search helps that are still available in the ABAP System?
Fortunately,
axelmohnen put in some effort and created an initial approach in the form of a generic Search Help Popup App. This app reads the standard SAP Search help and generates an abap2UI5 Popup from it, reminiscent of the previous SAP GUI popups:

abap2UI5 - Generic Search Help (Activated DDIC Objects)
As an example the demo above shows the search help for all activated Dictionary Objects. Check out the source code
here and the generic popup app
here. Of course, there is still some work to do, but even now it shows how easily you can work with generic typed tables and generic created popups in abap2UI5. Once again, the open-source project S-RTTI is used for realizing generic serialization. Thank you a lot
axelmohnen for your contribution and for sharing this idea.
11. Developer Code Challenge
During the
SAP ABAP Developer Challenge last May, abap2UI5 was used to create text adventure games based on
Axage by
enno.wulff. One particularly remarkable solution was developed by
jacques.nomssi, which includes images, text areas, input controls and many more. Look at this solution:

abap2UI5 - Open-Source Axage with Magical World in ABAP
For more information about this project, you can refer to his blog post about the "Magical World in ABAP"
here. He also has developed an abap2UI5 version of his
ABAP Scheme project. Thank you
jacques.nomssi
12. Demo & Sample Repository
Let's conclude the blog post with a complete demo where we can see that by combining a variety of controls, we are able to create apps with comprehensive functionality. This demo showcases tables, item navigation, rating indicators, pictures, text areas, search functionalities, and many more features:
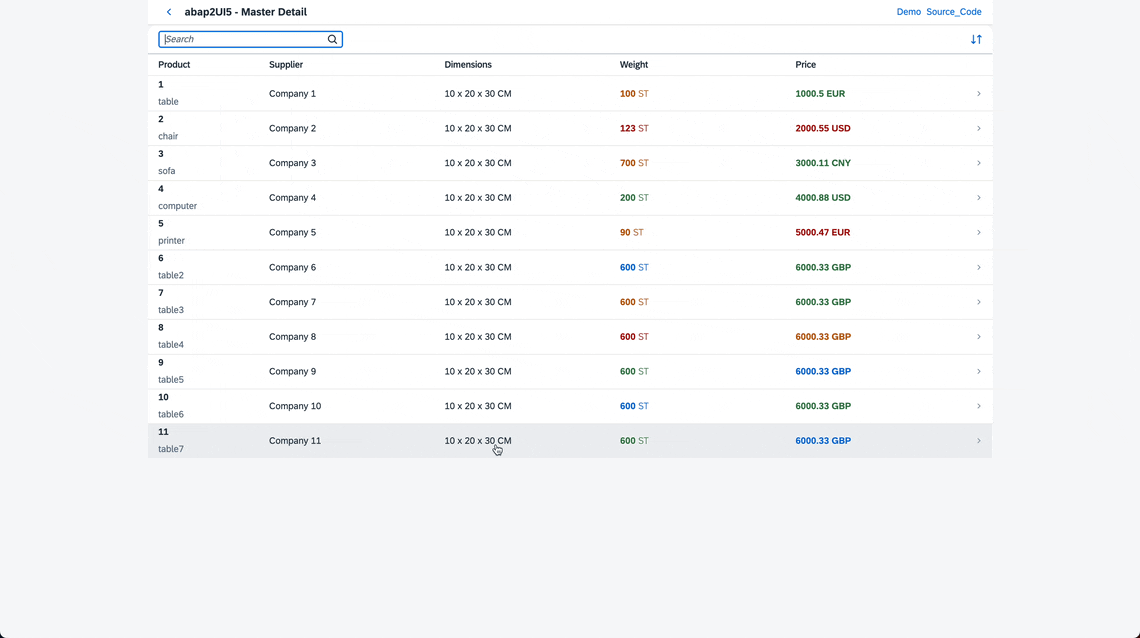
abap2UI5 - Complete Demo
The source code is a bit more complex, as you can see
here. However, it shows that creating more intricate apps is also possible. While abap2UI5 apps only implement a single method of an interface, you can easily also further encapsulate your apps to manage the growing logic of your views and controller. A big thank you to
Th0masMa for this great demo!
All code snippets and demos in the blog series are collected in the
abap2UI5 samples repository:

abap2UI5 - Samples
Feel free to explore it; the code snippets can be quickly copied & pasted and are ready-to-use for your own development.
13. Conclusion
This concludes the update on the functionalities of abap2UI5. Feel free to try out the new features in your own abap2UI5 apps, and should you encounter any problems, please don’t hesitate to open an
issue. Many of the new functionalities have been developed in response to issues raised in the past months. This proactive approach helps to identify areas where additional functionality is needed and it makes sense to extend abap2UI5.
I also encourage everyone to contribute to the project by submitting pull requests or expanding the samples repository. With abap2UI5, copying & pasting apps or functionalities is straightforward, so the more publicly available code snippets exist, the easier it gets to create apps with abap2UI5. Therefore, if you create your own app, also consider sharing it via a public git repository or as a pull request to the samples repository. Up until now, the number of samples has grown to nearly 100, which was only possible with the help of contributors from the ABAP community.
🙏
In the
next part, we will extend abap2UI5 with external libraries.
Thank you for reading! Your questions, comments and wishes for this project are always welcome, leave a comment or create an
issue.