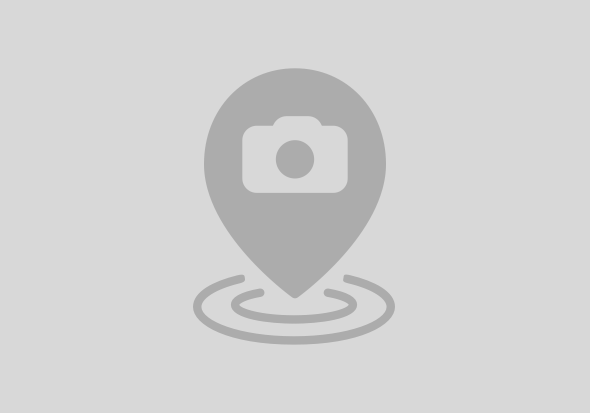
Demo Preview
sap.ui.define([
"sap/ui/core/UIComponent",
"sap/suite/ui/generic/template/extensionAPI/ReuseComponentSupport"
],
function (UIComponent, ReuseComponentSupport) {
"use strict";
return UIComponent.extend("demo.suresh.reusecomp.Component", {
metadata: {
manifest: "json",
library: "demoLibrary",
properties: {
/* Standard properties for reuse components */
uiMode: {
type: "string",
group: "standard"
},
semanticObject: {
type: "string",
group: "standard"
},
stIsAreaVisible: {
type: "boolean",
group: "standard"
},
/* Component specific properties */
customerID: {
type: "string",
group: "specific",
defaultValue: ""
}
}
},
init: function () {
//Transform this component into a reuse component for smart templates:
ReuseComponentSupport.mixInto(this);
//Defensive call of init of the super class:
( UIComponent.prototype.init || jQuery.noop ).apply(this, arguments);
}
});
}
);
setStIsAreaVisible: function (bIsAreaVisible) {
if (bIsAreaVisible !== this.getStIsAreaVisible()) {
this.setProperty("stIsAreaVisible", bIsAreaVisible);
}
},
setCustomerID: function (sCustomerID) {
if(sCustomerID !== this.getCustomerID()){
this.setProperty("customerID", sCustomerID);
this.getStIsAreaVisible () &&
this.getModel("northwind").metadataLoaded().then(this._setViewBinding.bind(this,sCustomerID));
}
},
_setViewBinding: function (sCustomerID) {
var oModel = this.getModel("northwind");
this._bindingPath = oModel.createKey("/Customers", {
CustomerID: sCustomerID
});
if (this._compView) {
this._compView.bindElement(this._bindingPath,{model:"northwind"});
}
}
onInit: function () {
this.getView().bindElement(this.getOwnerComponent().getBindingPath(),{model:"northwind"});
this.getOwnerComponent().setView(this.getView());
}
<mvc:View id="reuseCompView"
controllerName="demo.suresh.reusecomp.controller.View1"
xmlns:mvc="sap.ui.core.mvc" displayBlock="true"
xmlns="sap.m"
xmlns:f="sap.ui.layout.form">
<VBox id="vboxReuseComp">
<f:SimpleForm id="sfCustomerData"
editable="false"
layout="ResponsiveLayout"
title="Customer (Reuse Component)" >
<f:content>
<Label id="lblCustomerID" text="Customer ID"/>
<Text id="txtCustomerID" text="{CustomerID}" />
<Label id="lblCompanyName" text="Company Name" />
<Text id="txtCompanyName" text="{CompanyName}" />
<Label id="lblAddress" text="Address" />
<Text id="txtAddress" text="{Address}"/>
<Label id="lblRegion" text="Region"/>
<Text id="txtRegion" text="{Region}"/>
<Label id="lblCountry" text="Country"/>
<Text id="txtCountry" text="{Country}"/>
</f:content>
</f:SimpleForm>
</VBox>
</mvc:View>
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
46 | |
5 | |
5 | |
4 | |
3 | |
3 | |
3 | |
3 | |
2 | |
2 |