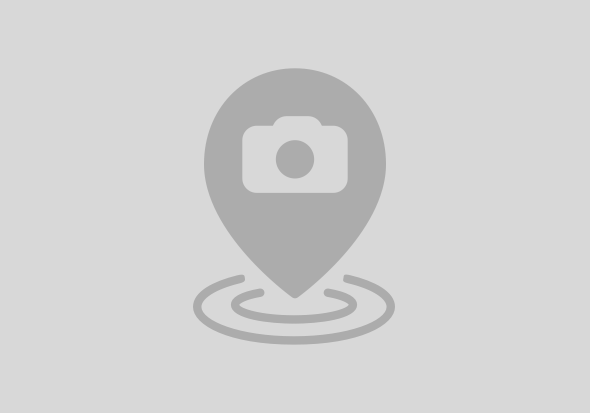
Fiori elements list report supports the prefilling of fields with default values when creating a new entity. Users will see a popup asking for input parameters. Based on these inputs, an event handler determines the default values to be pre-filled.
This functionality is specified through the use of annotations for prefilling fields, as illustrated below:
<Annotation Term="Common.DraftRoot">
<Record Type="Common.DraftRootType">
<PropertyValue Property="NewAction" String="com.sap.gateway.srvd.c_salesordermanage_sd.v0001.CreateWithSalesOrderType"/>
....
....
</Record>
</Annotation>
Source: Prefilling Fields When Creating a New Entity | UI5 Demo Kit
My aim is to achieve this functionality with CAP Node.js. While CAP Java documentation provides guidance on implementing this feature, there is currently no equivalent documentation for CAP Node.js.
Here goes the implementation. As you can see, it is fairly simple.
Service definition should follow the instructions provided in the CAP Java documentation.
using { my.booklist as db } from '../db/schema';
service BooklistService {
@odata.draft.enabled
@Common.DraftRoot.NewAction: 'BooklistService.createDraft'
entity Books as projection on db.Books actions {
action createDraft(in: many $self, title: String) returns Books;
};
}
The action must perform the following tasks:
import cds from '@sap/cds'
module.exports = class BooklistService extends cds.ApplicationService {
init() {
const { Books } = this.entities;
this.on('createDraft', Books, async (req) => {
//set initial data
const data = {
ID: cds.utils.uuid(),
title: req.data.title,
publisher: 'Test'
};
//create a draft
const book = await this.send({
query: INSERT.into(Books).entries(data),
event: "NEW",
});
//return the draft
return book;
})
return super.init();
}
}
According to the Q&A below, CAP Node.js does not provide draft API, so srv.send() has to be used instead.
In this blog, we explored how to prefill fields with default values when creating a new entity using CAP Node.js. By defining an action bound to the draft-enabled entity and implementing this action to set initial data and create a draft, developers can effectively prefill fields in their applications.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
10 | |
5 | |
4 | |
4 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 |