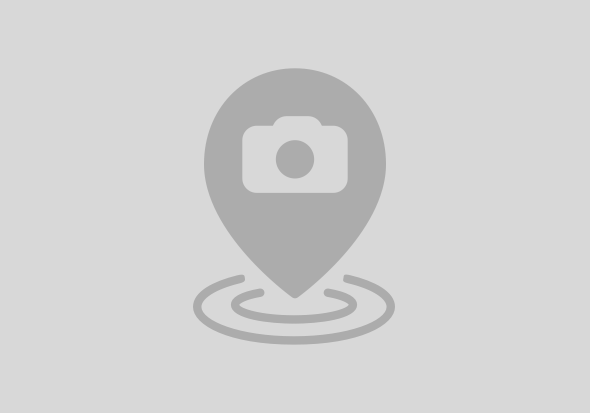
const express = require('express')
var parser = require('body-parser')
var session = require('express-session')
const expressApp = express(); // Created Express App
//URL PARSING Middleware
expressApp.use(parser.urlencoded({
extended: true
}))
//JSON PARSING Middleware
expressApp.use(parser.json());
// SESSION Middleware
expressApp.use(session({
secret: 'dsufhodsfhdiajdbadkjbaoodhdjbfadljqslvsoif', // Usually a long secret maintained in environment. For testing I maintained here
cookie: {
httpOnly: true, // Prevent reading the cookie from other than HTTP
maxAge: 150000, // Validity of session on milliseconds
domain: 'localhost' // Domain for cookie
},
resave: true,
saveUninitialized: false,
name: 'Session_ID' // Name on session id cookie on response
}))
//Login Route
expressApp.get("/login", function (req, res, next) {
console.log(req.session.id)
if (req.session.loggedin) { // IF already loggedin
res.status(200).send({
"message": "Session Authorized succesfully"
})
}
else {
const auth = new Buffer.from(req.headers.authorization.split(' ')[1], 'base64').toString().split(':'); // Getting user name and password from auth header
const user = auth[0];
const pass = auth[1];
if (user == "SAPCPI" && pass == '12345') { // For simplicity purpose
req.session.userid = req.body.userid // user id property
req.session.loggedin = true; // Logged In
res.status(200).send({
"message": "Logged In succesfully and session set for 1 hour"
})
}
else if (user == "SAPCPI_ADMIN" && pass == '98765') {
req.session.userid = req.body.userid
req.session.loggedin = true;
req.session.loggedinadmin = true;
res.status(200).send({
"message": "Logged In succesfully and session set for 1 hour"
})
}
else {
res.status(401).send({
"message": "Invalid Credentials. Please try again"
})
}
}
});
// Employee Details
expressApp.get('/getemployeedetails', function (req, res) {
console.log(req.headers.cookie)
if (req.session.loggedin) {
res.status(200).send({
"meessage": "Here you can found employee details"
})
} else {
res.status(401).send({
"message": "Unauthorized. Please login"
})
}
})
// Customer Details
expressApp.get('/getcustomerdetails', function (req, res) {
console.log(req.headers.cookie)
if (req.session.loggedin) {
res.status(200).send({
"meessage": "Here you can found vendor details"
})
} else {
res.status(401).send({
"message": "Unauthorized. Please login"
})
}
})
// Payment details
expressApp.get('/getpaymentdetails', function (req, res) {
if (req.session.loggedinadmin && req.session.loggedin) {
res.status(200).send({
"meessage": "Here you can found payment details"
})
} else {
if (req.session.loggedin) {
res.status(401).send({
"message": "You have no access to payment data.Contact Admin"
})
} else {
res.status(401).send({
"message": "Unauthorized. Please login"
})
}
}
})
expressApp.listen(8086, function () {
console.log("Server Listening on http://localhost:8086")
});
-----X-----
We can go in two ways.
1. If we go with "on Message Exchange," create a two-local process and call each separately. But as discussed, it will generate a new session on each hit.
2. If we go with "on Integration Flow," you can maintain a session for one user; if we pass that cookie to another, it will throw an error. We had to log out of this session and log in again for another service, which was not proper usage of a session.
How do we handle this? Let's share this in the second part of this blog.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
6 | |
5 | |
5 | |
4 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 |