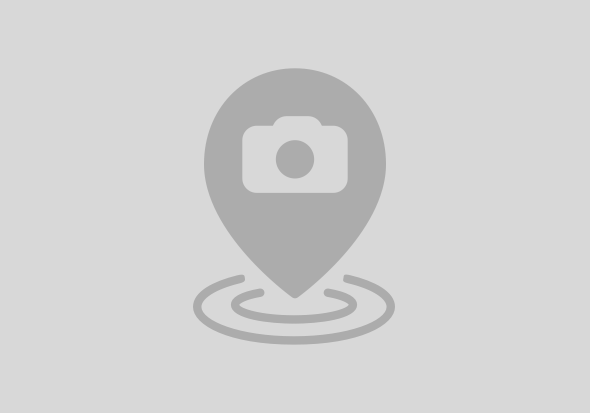
<%@ page import = "com.crystaldecisions.sdk.exception.SDKException,
com.crystaldecisions.sdk.framework.*,
com.crystaldecisions.sdk.occa.infostore.*,
com.crystaldecisions.sdk.properties.*,
com.crystaldecisions.sdk.plugin.desktop.server.*,
java.io.*,
java.util.*"
%><%
// ------------------------------
// How to use:
//
// Copy this jsp page into the folder C:\Program Files (x86)\SAP BusinessObjects\tomcat\webapps\AdminTools
//
// Modify the User Credentials Section to appropriate logon credentials for your system
// change fileToLogTo to whatever desired path you want the log file to be written to. The default is in the AdminTools folder referenced above
// Change pathToInputFRS and pathToOutputFRS to the location of your Input and Output FRS
// If you set Run_In_Test_Mode to true this sample will run in Demo mode and will not delete files
// If you set Run_In_Test_Mode to false this sample will run in delete mode and will delete files
// Run this by opening a new browser and going to http://myServer:8080/AdminTools/scanFRS.jsp
// ------------------------------
Boolean Run_In_Test_Mode = true; // true = demo mode false = delete mode
String pathToInputFRS = "C:\\Program Files (x86)\\SAP BusinessObjects\\SAP BusinessObjects Enterprise XI 4.0\\FileStore\\Input";
String pathToOutputFRS = "C:\\Program Files (x86)\\SAP BusinessObjects\\SAP BusinessObjects Enterprise XI 4.0\\FileStore\\Output";
String fileToLogTo = "C:\\Program Files (x86)\\SAP BusinessObjects\\tomcat\\webapps\\AdminTools\\TestOutput.txt";
// User Credentials
String username = "Administrator";
String password = "Password1";
String cmsname = "localhost";
String authType = "secEnterprise";
IEnterpriseSession enterpriseSession = null;
IInfoStore boInfoStore;
enterpriseSession = CrystalEnterprise.getSessionMgr().logon(username, password, cmsname, authType);
boInfoStore = (IInfoStore)enterpriseSession.getService("", "InfoStore");
out.println("Starting </BR>");
writeToLog("Starting Sample", fileToLogTo);
// The logic used is:
// - Is there a file? If not, is there a sub-folder.
// - If there is a sub-folder - then go into it
// - If there is a file - then look at the last folder name - that will be the SI_ID
// - Check if an object with that SI_ID exists. If it does - all is well - if not - flag for deletion
// - The deletion will go up folder by folder until one of three conditions occur.
// - 1. A file exists in the folder.
// - 2. More than 1 sub-folder
// - 3. The name of the current folder is either Input, or Output
Boolean isDoneInput = CheckAndDelete(Run_In_Test_Mode, boInfoStore, fileToLogTo, pathToInputFRS, "Input");
Boolean isDoneOutput = CheckAndDelete(Run_In_Test_Mode, boInfoStore, fileToLogTo, pathToOutputFRS, "Output");
writeToLog("Finished Sample </BR>", fileToLogTo);
out.println("Job Completed");
%>
<%!
// Helper Methods
public void writeToLog(String msg, String logFilePath) {
try {
// Set up Logging File
FileOutputStream FSout;
PrintStream pStream; // declare a print stream object
FSout = new FileOutputStream(logFilePath, true); // Append
pStream = new PrintStream(FSout);
pStream.println(msg);
pStream.close();
} catch (IOException e) {
//error writing to log
}
}
public Boolean CheckAndDelete(Boolean doDelete, IInfoStore boInfoStore, String fileToLogTo, String currentFolderPath, String currentFolderName) {
// Loop through all the files / subdirectories in the current directory
int subDirCount = 0;
Boolean isOrphan = false;
Boolean checkFiles = false;
try {
File currentDir = new File(currentFolderPath);
File[] listFiles = currentDir.listFiles();
for (File file : listFiles)
{
// Is this a sub-directory
if (file.isDirectory())
{
// Do the recursive check into the directory. If it returns true, then the sub-folder is now clear and can be deleted.
if (CheckAndDelete(doDelete, boInfoStore, fileToLogTo, file.getCanonicalPath(), file.getName()) == true) {
// Are we in demo mode
if (doDelete == false) {
file.delete();
}
}
} else {
// Have we already checked the files for this directory.
if (checkFiles == false) {
// Since there are files - that means this is an infoobject and we need to check it.
// The current directory name is the SI_ID of the infoobject, so query for that.
IInfoObjects boInfoObjects = (IInfoObjects)boInfoStore.query("Select * from CI_INFOOBJECTS, CI_APPOBJECTS, CI_SYSTEMOBJECTS where SI_ID = " + currentFolderName);
// Did we get a result
if (boInfoObjects.size() == 0) {
isOrphan = true;
}
// We have already checked this object - no need to check it again.
checkFiles = true;
}
// If we verified that this is an orphan - delete the file
if (isOrphan == true) {
writeToLog("Deleting file " + file.getCanonicalPath() + " from Orphaned Infoobject with SI_ID " + currentFolderName, fileToLogTo);
// Are we in demo mode
if (doDelete == false) {
file.delete();
}
}
}
}
} catch (IOException e) {
e.printStackTrace();
} catch (SDKException ex) {
ex.printStackTrace();
}
return (isOrphan);
}
%>
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
17 | |
11 | |
7 | |
7 | |
7 | |
7 | |
6 | |
6 | |
6 | |
6 |