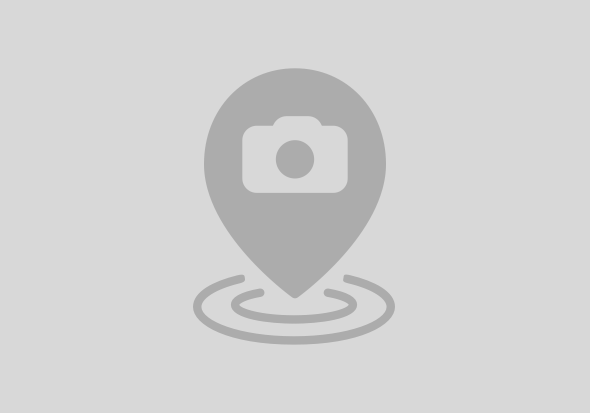
<f:SimpleForm id="SalesOrder" title="Sales Order {SalesOrder}" editable="true" Layout="ResponsiveGridLayout" singleContainerFullSize="false">
<f:toolbar>
....
</f:toolbar>
<f:content>
<Label text="Text"/>
<Input value="{SalesOrder_Text}" editable="{detailView>/editMode}"/>
<Label text="Net Amount"/>
<Input value="{NetAmountInTransactionCurrency}" editable="false"/>
<Label text="Gross Amount"/>
<Input value="{GrossAmountInTransacCurrency}" editable="false"/>
</f:content>
</f:SimpleForm>
onSave: function(oEvent) {
this._update();
},
_update: function() {
var sPath = that.getView().getBindingContext().getPath();
var oSalesOrder = that.getView().getBindingContext().getObject();
this._updateSalesOrder(sPath, oSalesOrder)
.then(function() {
MessageToast.show("Success...");
})
.catch(function(oError) {
//Error handling...
});
},
_updateSalesOrder: function(sPath, oSalesOrder, bForceUpdate) {
var oDataModel = this.getView().getModel();
return new Promise(function(resolve, reject) {
oDataModel.update(sPath, oPlan, {
success: resolve,
error: reject
});
});
},
.catch(function(oError) {
// Error handling
//open Dialog if Precondition failed
if (oError === "412") {
that._openDialog();
}
});
_openDialog: function() {
var that = this;
var dialog = new Dialog({
title: 'Confirm',
type: 'Message',
content: new Text({
text: 'There is a more recent version available. Do you want to refresh or overwrite the backend entry?'
}),
beginButton: new Button({
text: 'Overwrite',
press: function() {
that._update(true);
dialog.close();
}
}),
endButton: new Button({
text: 'Refresh',
press: function() {
//Refresh entity....
dialog.close();
}
}),
afterClose: function() {
dialog.destroy();
}
});
dialog.open();
},
_update: function(bForceUpdate) {
var that = this;
var sPath = that.getView().getBindingContext().getPath();
var oModel = that.getView().getModel();
var oSalesOrder = that.getView().getBindingContext().getObject();
this._updateSalesOrder(sPath, oSalesOrder, bForceUpdate)
.then(function() {
MessageToast.show("Success...");
})
.catch(function(oError) {
// Error handling
//open Dialog if Precondition failed
if (oError === "412") {
that._openDialog();
}
});
},
_updateSalesOrder: function(sPath, oSalesOrder, bForceUpdate) {
var oDataModel = this.getView().getModel();
var oPromise = new Promise(function(resolve, reject) {
var mParameters = {
success: resolve,
error: function(oError) {
reject(oError.statusCode);
}
};
if (bForceUpdate) {
mParameters.eTag = "*";
}
oDataModel.update(sPath, oSalesOrder, mParameters);
});
return oPromise;
},
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
11 | |
10 | |
7 | |
7 | |
6 | |
6 | |
5 | |
5 | |
5 | |
5 |