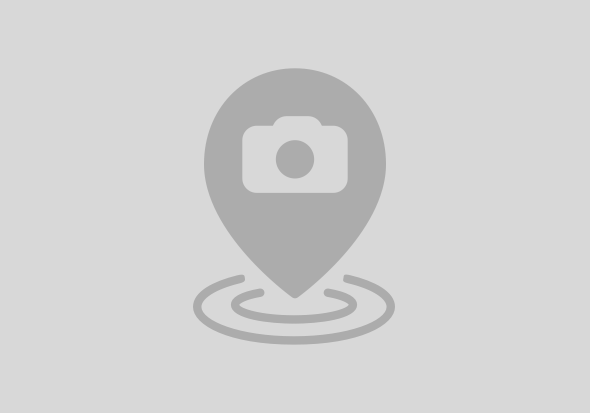
TYPES: BEGIN OF lt_head,
docnr TYPE n LENGTH 10,
gjahr TYPE gjahr,
END OF lt_head,
BEGIN OF lt_position,
docnr TYPE n LENGTH 10,
gjahr TYPE gjahr,
posnr TYPE n LENGTH 3,
END OF lt_position.
DATA: li_head TYPE STANDARD TABLE OF lt_head
WITH HEADER LINE,
li_positions TYPE STANDARD TABLE OF lt_position
WITH HEADER LINE,
li_positions_sorted TYPE SORTED TABLE OF lt_position
WITH NON-UNIQUE KEY docnr gjahr posnr
WITH HEADER LINE.
START_MEASUREMENT 'SORT'.
SORT li_positions BY docnr gjahr posnr.
END_MEASUREMENT 'SORT'.
START_MEASUREMENT 'LOOP WHERE'.
LOOP AT li_head.
LOOP AT li_positions WHERE docnr = li_head-docnr
AND gjahr = li_head-gjahr.
ENDLOOP.
ENDLOOP.
END_MEASUREMENT 'LOOP WHERE'.
START_MEASUREMENT 'LOOP WHERE SORTED TABLE'.
LOOP AT li_head.
LOOP AT li_positions_sorted WHERE docnr = li_head-docnr
AND gjahr = li_head-gjahr.
ENDLOOP.
ENDLOOP.
END_MEASUREMENT 'LOOP WHERE SORTED TABLE'.
START_MEASUREMENT 'BINARY SEARCH'.
LOOP AT li_head.
READ TABLE li_positions WITH KEY docnr = li_head-docnr
gjahr = li_head-gjahr
BINARY SEARCH.
IF sy-subrc = 0.
LOOP AT li_positions FROM sy-tabix.
IF li_positions-docnr <> li_head-docnr OR
li_positions-gjahr <> li_head-gjahr.
EXIT.
ENDIF.
ENDLOOP.
ENDIF.
ENDLOOP.
END_MEASUREMENT 'BINARY SEARCH'.
START_MEASUREMENT 'BINARY SEARCH SORTED TABLE'.
LOOP AT li_head.
READ TABLE li_positions_sorted WITH KEY docnr = li_head-docnr
gjahr = li_head-gjahr
BINARY SEARCH.
IF sy-subrc = 0.
LOOP AT li_positions_sorted FROM sy-tabix.
IF li_positions_sorted-docnr <> li_head-docnr OR
li_positions_sorted-gjahr <> li_head-gjahr.
EXIT.
ENDIF.
ENDLOOP.
ENDIF.
ENDLOOP.
END_MEASUREMENT 'BINARY SEARCH SORTED TABLE'.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
4 | |
3 | |
3 | |
2 | |
2 | |
2 | |
2 | |
1 | |
1 | |
1 |