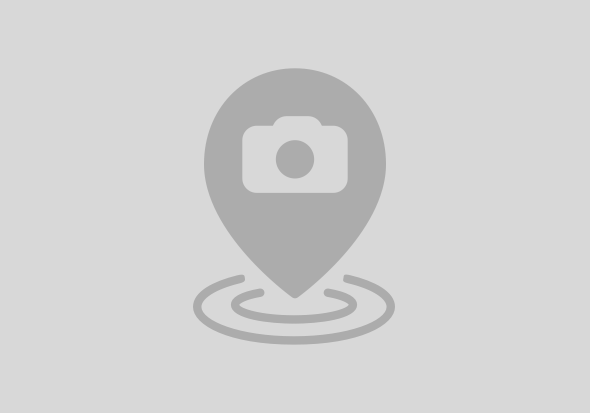
With 7.40, SP05 the first version of the iteration operator FOR was introduced. You can use it in constructor expressions with VALUE and NEW for so called table comprehensions, as e.g.
DATA(itab2) = VALUE t_itab2( FOR wa IN itab1 WHERE ( col1 < 30 )
( col1 = wa-col2 col2 = wa-col3 ) ).
This is an expression enabled version of LOOP AT itab. It didn't take long to ask also for expression enabled versions of DO and WHILE (couldn't stand them in otherwise expression enabled examples any more ...).
Therefore, with 7.40, SP08 we also offer conditional iterations with FOR:
... FOR i = ... [THEN expr] UNTIL|WHILE log_exp ...
You can use FOR in constructor expressions with VALUE and NEW in order to create new internal tables, e.g.:
TYPES:
BEGIN OF line,
col1 TYPE i,
col2 TYPE i,
col3 TYPE i,
END OF line,
itab TYPE STANDARD TABLE OF line WITH EMPTY KEY.
DATA(itab) = VALUE itab(
FOR j = 11 THEN j + 10 UNTIL j > 40
( col1 = j col2 = j + 1 col3 = j + 2 ) ).
gives
COL1 | COL2 | COL3 |
11 | 12 | 13 |
21 | 22 | 23 |
31 | 32 | 33 |
Neat, isn't it?
But we don't want to construct internal tables only. Now that we have all kinds of iterations available with FOR, we want to construct arbitrary types. And that's where the new constructor operator REDUCE comes in.
... REDUCE type(
INIT result = start_value
...
FOR for_exp1
FOR for_exp2
...
NEXT ...
result = iterated_value
... ) ...
While VALUE and NEW expressions can include FOR expressions, REDUCE must include at least one FOR expression. You can use all kinds of FOR expressions in REDUCE:
Let's reduce an internal table:
DATA itab TYPE STANDARD TABLE OF i WITH EMPTY KEY.
itab = VALUE #( FOR j = 1 WHILE j <= 10 ( j ) ).
DATA(sum) = REDUCE i( INIT x = 0 FOR wa IN itab NEXT x = x + wa ).
First, the table is filled with VALUE and FOR and then it is reduced with REDUCE to the sum of its contents. Note that there is no THEN used to construct the table. If THEN is not specified explicitly, implicitly THEN j = j + 1 is used. Be also aware, that you can place any expression behind THEN, including method calls. You only have to make sure that the end condition is reached within maximal program run time.
Now let's reduce the values of a conditional iteration into a string:
DATA(result) =
REDUCE string( INIT text = `Count up:`
FOR n = 1 UNTIL n > 10
NEXT text = text && | { n }| ).
The result is
Count up: 1 2 3 4 5 6 7 8 9 10
These simple examples show the principle. Now imagine, what you can do by mixing REDUCE with all the other expression enabled capabilities. I only say nested REDUCE and VALUE operators ...
To conclude I show a cunning little thing that I use in some of my documentation examples:
TYPES outref TYPE REF TO if_demo_output.
DATA(output) =
REDUCE outref( INIT out = cl_demo_output=>new( )
text = `Count up:`
FOR n = 1 UNTIL n > 11
NEXT out = out->write( text )
text = |{ n }| ).
output->display( ).
I reduced the values of an iteration into the output list of a display object, oh my ...
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
4 | |
3 | |
2 | |
2 | |
2 | |
2 | |
2 | |
1 | |
1 | |
1 |