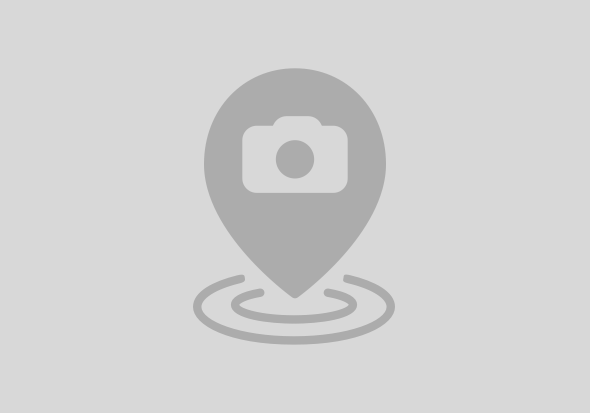
class ZCL_CIRCLE definition
public
final
create public .
public section.
methods CONSTRUCTOR
importing
!IV_RADIUS type FLOAT .
methods GET_AREA
returning
value(RV_RESULT) type FLOAT .
protected section.
private section.
data RADIUS type FLOAT .
ENDCLASS.
CLASS ZCL_CIRCLE IMPLEMENTATION.
method CONSTRUCTOR.
radius = iv_radius.
endmethod.
method GET_AREA.
CONSTANTS: pai TYPE float value '3.14'.
rv_result = pai * radius * radius.
endmethod.
ENDCLASS.
class ZCL_RECTANGLE definition
public
final
create public .
public section.
methods CONSTRUCTOR
importing
!IV_HEIGHT type FLOAT
!IV_WIDTH type FLOAT .
methods GET_AREA
returning
value(RV_RESULT) type FLOAT .
protected section.
private section.
data HEIGHT type FLOAT .
data WIDTH type FLOAT .
ENDCLASS.
CLASS ZCL_RECTANGLE IMPLEMENTATION.
method CONSTRUCTOR.
height = iv_height.
width = iv_width.
endmethod.
method GET_AREA.
rv_result = width * height.
endmethod.
ENDCLASS.
REPORT ZCALCULATE1.
TYPES: BEGIN OF ty_shape,
shape TYPE REF TO object,
END OF ty_shape.
TYPES: tt_shape TYPE STANDARD TABLE OF ty_shape.
DATA: lt_shape TYPE tt_shape,
lv_result TYPE float.
START-OF-SELECTION.
data(lo_circle) = new zcl_circle( 1 ).
data(entry) = value ty_shape( shape = lo_circle ).
APPEND entry TO lt_shape.
data(lo_circle2) = new zcl_circle( 1 ).
entry = value #( shape = lo_circle2 ).
APPEND entry TO lt_shape.
data(lo_rectangle) = new zcl_rectangle( iv_width = 1 iv_height = 2 ).
entry = value #( shape = lo_rectangle ).
APPEND entry TO lt_shape.
LOOP AT lt_shape ASSIGNING FIELD-SYMBOL(<shape>).
IF <shape>-shape IS INSTANCE OF zcl_circle.
lv_result = lv_result + cast zcl_circle( <shape>-shape )->get_area( ).
ELSEIF <shape>-shape IS INSTANCE OF zcl_rectangle.
lv_result = lv_result + cast zcl_rectangle( <shape>-shape )->get_area( ).
ELSE.
"reserved for other shape type in the future
ENDIF.
ENDLOOP.
WRITE: / lv_result.
interface ZIF_SHAPE
public .
methods GET_AREA
returning
value(RV_RESULT) type FLOAT .
endinterface.
REPORT ZCALCULATE2.
data: lv_result TYPE float.
data(lo_container) = new cl_object_collection( ).
data(lo_circle) = new zcl_circle( 1 ).
lo_container->add( lo_circle ).
data(lo_circle2) = new zcl_circle( 1 ).
lo_container->add( lo_circle2 ).
data(lo_rectangle) = new zcl_rectangle( iv_width = 1 iv_height = 2 ).
lo_container->add( lo_rectangle ).
data(lo_iterator) = lo_container->get_iterator( ).
WHILE lo_iterator->has_next( ).
data(lo_shape) = cast ZIF_SHAPE( lo_iterator->get_next( ) ).
lv_result = lv_result + lo_shape->get_area( ).
ENDWHILE.
WRITE: / lv_result.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
4 | |
3 | |
3 | |
2 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 |