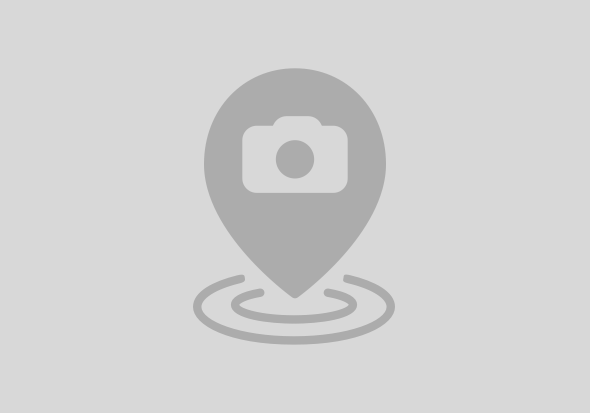
<Document>
<Name>
Document_Name
</Name>
<Content>
Document_content_here
</Content>
</Document>
{
"Name" : "Document_Name",
"Content" : "Document_content_here"
}
interface ZIF_CK_DATA_CARRIER
public .
types: "! Coordinates of weather station
begin of coord,
lon type p length 5 decimals 2,
lat type p length 5 decimals 2,
end of coord,
"! Weather conditions
begin of weather_line,
id type i,
main type string,
description type string,
icon type char10,
end of weather_line,
tt_weather type standard table of weather_line with default key,
begin of request_line,
coord type coord,
weather type tt_weather,
base type string,
"! Main weather data
begin of main,
temp type p length 5 decimals 2,
pressure type i,
humidity type i,
temp_min type p length 5 decimals 2,
temp_max type p length 5 decimals 2,
end of main,
"! Visibility in meters
visibility type i,
"! Wind conditions
begin of wind,
speed type p length 5 decimals 2,
deg type i,
end of wind,
"! Clouds state (percentage)
begin of clouds,
all type i,
end of clouds,
"! Unix datetime of measurement
dt type string,
"! Some system messages and sunrise and sunset information
begin of sys,
type type i,
id type i,
message type p length 5 decimals 4,
country type char10,
sunrise type i,
sunset type i,
end of sys,
"! Unique ID of the city/station
id type string,
"! City/station name
name type string,
"! HTTP code returned
cod type i,
end of request_line.
methods: get_data returning value(r_data_table) type request_line,
set_data importing i_value type string.
endinterface.
class zcl_ck_http_client definition
public
final
create public .
public section.
interfaces: zif_ck_data_carrier.
types: begin of location,
country type char03,
city type char40,
end of location.
methods:
"! <p class="shorttext synchronized" lang="en"></p>
"! Create object, send request and parse JSON data
"! @parameter i_location | <p class="shorttext synchronized" lang="en"></p>
"! @parameter i_auth_key | <p class="shorttext synchronized" lang="en"></p>
constructor importing i_location type location i_auth_key type string,
"! <p class="shorttext synchronized" lang="en"></p>
"! Returns cityname
"! @parameter r_city | <p class="shorttext synchronized" lang="en"></p>
get_city returning value(r_city) type location-city,
"! <p class="shorttext synchronized" lang="en"></p>
"! Returns country name
"! @parameter r_country | <p class="shorttext synchronized" lang="en"></p>
get_country returning value(r_country) type location-country,
"! <p class="shorttext synchronized" lang="en"></p>
"! Returns http_client.
"! @parameter r_http_client | <p class="shorttext synchronized" lang="en"></p>
get_http_client returning value(r_http_client) type ref to if_http_client.
protected section.
private section.
data: request_line type zif_ck_data_carrier~request_line,
city type location-city,
country type location-country,
http_client type ref to if_http_client.
methods: "! <p class="shorttext synchronized" lang="en"></p>
"! Construct url address for HTTP Client
"! @parameter i_country | Country for which data is fetched <p class="shorttext synchronized" lang="en"></p>
"! @parameter i_city | City for which data is fethced <p class="shorttext synchronized" lang="en"></p>
"! @parameter i_auth_key | Unique authorization key for user <p class="shorttext synchronized" lang="en"></p>
"! @parameter r_url_address | <p class="shorttext synchronized" lang="en"></p>
get_url_address importing i_country type location-country
i_city type location-city
i_auth_key type string
returning value(r_url_address) type string.
endclass.
class zcl_ck_http_client implementation.
method zif_ck_data_carrier~get_data.
r_data_table = request_line.
endmethod.
method get_url_address.
r_url_address = |http://api.openweathermap.org/data/2.5/weather?q={ i_city },{ i_country }&appid={ i_auth_key }|.
endmethod.
method constructor.
cl_http_client=>create_by_url( exporting url = get_url_address( i_country = i_location-country
i_city = i_location-city
i_auth_key = i_auth_key ) " URL
importing client = http_client " HTTP Client Abstraction
exceptions argument_not_found = 1
plugin_not_active = 2
internal_error = 3
others = 4 ).
if sy-subrc <> 0.
raise exception type cx_sy_ref_creation.
endif.
http_client->send( ).
http_client->receive( ).
zif_ck_data_carrier~set_data( i_value = http_client->response->get_cdata( ) ).
city = i_location-city.
country = i_location-country.
endmethod.
method get_city.
r_city = city.
endmethod.
method get_country.
r_country = country.
endmethod.
method get_http_client.
r_http_client = http_client.
endmethod.
method zif_ck_data_carrier~set_data.
/ui2/cl_json=>deserialize( EXPORTING json = i_value pretty_name = /ui2/cl_json=>pretty_mode-low_case CHANGING data = request_line ).
endmethod.
endclass.
*"* use this source file for your ABAP unit test classes
class ltcl_object_creation definition final for testing
duration short
risk level harmless.
private section.
methods:
http_object_creator for testing raising cx_static_check.
endclass.
class ltcl_object_creation implementation.
method http_object_creator.
cl_abap_unit_assert=>assert_not_initial( exporting act = new zcl_ck_http_client( i_location = value #( city = 'London' country = 'UK' )
i_auth_key = 'YourAuthKeyHere' )->zif_ck_data_carrier~get_data( ) ).
endmethod.
endclass.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
2 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 |