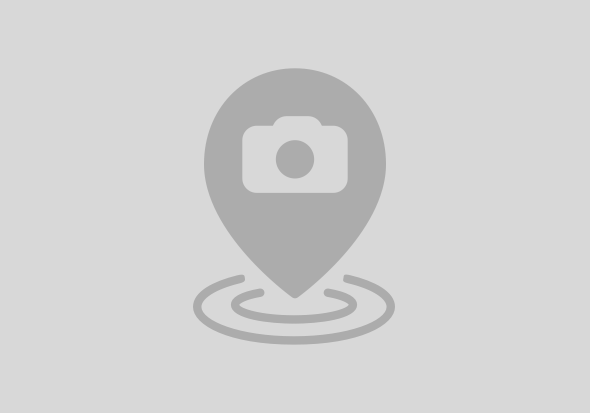
Structure of table ZTC8A005_SAMPLE (the separate entity) | ||
---|---|---|
Field ID | Data Type | Comment |
MANDT | MANDT | Key |
ENTITY_GUID | CHAR32 | Key |
ENTITY_PARAM1 | CHAR10 | Sample of short char-field |
ENTITY_PARAM2 | NUMC10 | Sample of numc-field |
ENTITY_PARAM3 | SYUZEIT | Sample of time-field |
ENTITY_PARAM4 | SYDATUM | Sample of date-field |
ENTITY_PARAM5 | TIMESTAMP | Sample of TIMESTAMP (dec-field) |
ENTITY_PARAM6 | INT4 | Sample of integer-field |
Picture 1. Attributes of update function module for table ZTC8A005_SAMPLE
Picture 2. Import parameters pass by value for update function
Picture 3. Separate table type for table ZTC8A005_SAMPLE
FUNCTION z_c8a_005_demo_upd_sample.
*"----------------------------------------------------------------------
*" IMPORTING
*" VALUE(IV_UPDKZ) TYPE UPDKZ DEFAULT 'M'
*" VALUE(IT_SAMPLE) TYPE ZTC8A005_SAMPLE_TAB_TYPE
*"----------------------------------------------------------------------
DATA lc_modify_tab TYPE updkz VALUE 'M'.
DATA lc_upd_tab TYPE updkz VALUE 'U'.
DATA lc_del_tab TYPE updkz VALUE 'D'.
IF it_sample IS INITIAL.
EXIT.
ENDIF.
CASE iv_updkz.
WHEN lc_modify_tab.
MODIFY ztc8a005_sample FROM TABLE it_sample.
WHEN lc_upd_tab.
UPDATE ztc8a005_sample FROM TABLE it_sample.
WHEN lc_del_tab.
DELETE ztc8a005_sample FROM TABLE it_sample.
WHEN OTHERS.
ENDCASE.
ENDFUNCTION.
DATA lt_sample_tab TYPE STANDARD TABLE OF ztc8a005_sample.
lt_sample_tab = VALUE #(
( entity_guid = 'FUNC_GUID_MOD' entity_param1 = 'CHAR10' entity_param2 = '0504030201' )
( entity_guid = 'FUNC_GUID2_MOD' entity_param1 = '2CHAR10' entity_param2 = '0102030405' )
( entity_guid = 'FUNC_GUID2_DEL' entity_param1 = '2CHAR10' entity_param2 = '777909034' ) ).
CALL FUNCTION 'Z_C8A_005_DEMO_UPD_SAMPLE'
IN UPDATE TASK
EXPORTING
it_sample = lt_sample_tab.
CALL FUNCTION 'BAPI_TRANSACTION_COMMIT'
EXPORTING
wait = abap_true.
DATA lc_db_tab_sample TYPE tabname VALUE 'ZTC8A005_SAMPLE'.
DATA lt_sample_tab TYPE STANDARD TABLE OF ztc8a005_sample.
lt_sample_tab = VALUE #(
( entity_guid = 'FUNC_GUID_MOD' entity_param1 = 'CHAR10' entity_param2 = '0504030201' )
( entity_guid = 'FUNC_GUID2_MOD' entity_param1 = '2CHAR10' entity_param2 = '0102030405' )
( entity_guid = 'FUNC_GUID2_DEL' entity_param1 = '2CHAR10' entity_param2 = '777909034' ) ).
NEW zcl_c8a005_save2db(
)->save2db( iv_tabname = lc_db_tab_sample
it_tab_content = lt_sample_tab )->do_commit_if_any( ).
DATA lc_db_tab_sample TYPE tabname VALUE 'ZTC8A005_SAMPLE'.
DATA lt_sample_tab TYPE STANDARD TABLE OF ztc8a005_sample.
DATA lt_sample_empty_tab TYPE STANDARD TABLE OF ztc8a005_sample.
DATA lt_head_tab TYPE STANDARD TABLE OF ztc8a005_head.
DATA lt_item_tab TYPE STANDARD TABLE OF ztc8a005_item.
DATA lv_ts TYPE timestamp.
DATA lo_saver_anytab TYPE REF TO zcl_c8a005_save2db.
GET TIME STAMP FIELD lv_ts.
lt_sample_tab = VALUE #(
( entity_guid = 'ANY_GUID_MOD' entity_param1 = 'CHAR10' entity_param2 = '0504030201'
entity_param3 = sy-uzeit entity_param4 = sy-datum entity_param5 = lv_ts )
( entity_guid = 'ANY_GUID2_MOD' entity_param1 = '2CHAR10' entity_param2 = '0102030405'
entity_param3 = sy-uzeit entity_param4 = sy-datum entity_param5 = lv_ts )
( entity_guid = 'ANY_GUID2_DEL' entity_param1 = '2CHAR10' entity_param2 = '777909034'
entity_param3 = sy-uzeit entity_param4 = sy-datum entity_param5 = lv_ts )
).
lt_head_tab = VALUE #(
( head_guid = 'ANY_GUID_UPD' head_param1 = 'ANY_GUID_ADD' head_param2 = '9988776655'
head_param3 = sy-uzeit head_param4 = sy-datum head_param5 = lv_ts )
( head_guid = 'ANY_GUID2_UPD' head_param1 = 'ANY_GUID2_ADD' head_param2 = '9988776655'
head_param3 = sy-uzeit head_param4 = sy-datum head_param5 = lv_ts )
( head_guid = 'ANY_GUID_DEL' head_param1 = 'ANY_GUID_ADD' head_param2 = '9988774444'
head_param3 = sy-uzeit head_param4 = sy-datum head_param5 = lv_ts )
( head_guid = 'ANY_GUID2_DEL' head_param1 = 'ANY_GUID2_ADD' head_param2 = '9988774444'
head_param3 = sy-uzeit head_param4 = sy-datum head_param5 = lv_ts )
).
lt_item_tab = VALUE #(
( head_guid = 'ANY_GUID_UPD' item_guid = 'ANY_ITEM_GUID_ADD' item_param1 = '2CHAR10' item_param2 = '9988776655'
item_param3 = sy-uzeit item_param4 = sy-datum item_param5 = lv_ts )
( head_guid = 'ANY_GUID2_UPD' item_guid = 'ANY_ITEM_GUID2_ADD' item_param1 = '2CHAR10'
item_param3 = sy-uzeit item_param4 = sy-datum item_param5 = lv_ts )
( head_guid = 'ANY_GUID_DEL' item_guid = 'ANY_ITEM_GUID_ADD' item_param2 = '9988776655'
item_param3 = sy-uzeit item_param4 = sy-datum item_param5 = lv_ts )
( head_guid = 'ANY_GUID2_DEL' item_guid = 'ANY_ITEM_GUID2_ADD' item_param1 = '2CHAR10'
item_param3 = sy-uzeit item_param4 = sy-datum item_param5 = lv_ts )
).
CREATE OBJECT lo_saver_anytab.
lo_saver_anytab->save2db( EXPORTING iv_tabname = lc_db_tab_sample
it_tab_content = lt_sample_tab ).
lo_saver_anytab->save2db( EXPORTING iv_tabname = 'ZTC8A005_HEAD'
it_tab_content = lt_head_tab ).
lo_saver_anytab->save2db( EXPORTING iv_tabname = 'ZTC8A005_ITEM'
it_tab_content = lt_item_tab ).
CLEAR lt_sample_empty_tab.
lo_saver_anytab->save2db( EXPORTING iv_tabname = lc_db_tab_sample
it_tab_content = lt_sample_empty_tab ).
" database changes are to be after commit-command (which is in method do_commit_if_any )
" empty table does not take into account while commit command
lo_saver_anytab->do_commit_if_any( ).
DATA lt_sample_tab TYPE STANDARD TABLE OF ztc8a005_sample.
lt_sample_tab = VALUE #(
( entity_guid = 'ANY_SIMPL_GUID_MOD' entity_param1 = 'CHAR10' entity_param2 = '0504030201' )
( entity_guid = 'ANY_SIMPL_GUID2_MOD' entity_param1 = '2CHAR10' entity_param2 = '0102030405' )
( entity_guid = 'ANY_SIMPL_GUID2_DEL' entity_param1 = '2CHAR10' entity_param2 = '777909034' )
).
CREATE OBJECT lo_saver_anytab.
lo_saver_anytab->save2db( EXPORTING it_tab_content = lt_sample_tab )->do_commit_if_any( ).
DATA lt_sample01 TYPE ztc8a005_sample_tab_type. " by table type
" recomended:
DATA lt_sample02 TYPE STANDARD TABLE OF ztc8a005_sample. " dynamical typing by STANDARD TABLE OF DB_tabname
" Could by on the basis of interface
INTERFACE ltc_interface4type.
TYPES ts_data TYPE ztc8a005_sample.
TYPES tt_data TYPE STANDARD TABLE OF ts_data WITH DEFAULT KEY.
ENDINTERFACE.
DATA lt_sample03 TYPE STANDARD TABLE OF ltc_interface4type=>ts_data.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
3 | |
2 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 |