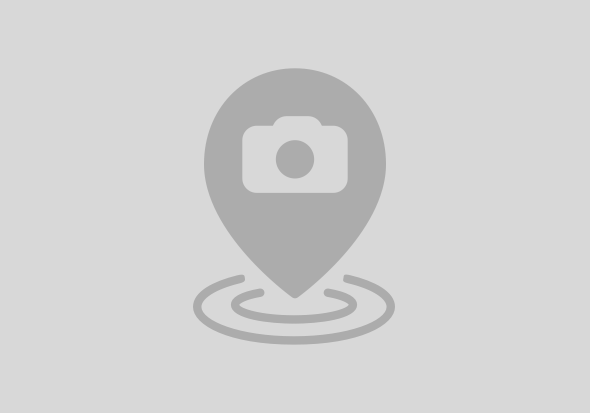
Ever since the CL_SALV_TABLE class was released, one question was ever present - how to make it editable? For years the answer lied in taking advantage of the inheritance of the CL_SALV_TABLE, as presented in this post: http://zevolving.com/2008/12/salv-table-10-editable-salv-model-overcome-the-restriction-of-salv-mode....
I was among those using this method. But then the Release 756 came. And suddenly, all ALVs using it suddenly started reported error after error. I dived in and investigated the issue. To my surprise, I discovered that the very basis of this "edit-hack" was gone. The CL_SALV_TABLE class was no longer in the inheritance tree which enabled it to work!
And so followed combing through the provided interfaces in an effort to find a new way. And lo and behold! A way I indeed found.
In this post, I will share with you how I achieved CL_SALV_TABLE editable upon button-press, complete with F4 references and save function in post-release 756 environment.
Editable CL_SALV_TABLE
cl_salv_table=>factory(
EXPORTING
list_display = abap_false
IMPORTING
r_salv_table = o_salv
CHANGING
t_table = t_data ).
DATA lt_cols TYPE REF TO cl_salv_columns_table.
DATA ls_col_grbew TYPE REF TO cl_salv_column.
DATA lv_grbew TYPE lvc_fname.
DATA ls_grbew_ref TYPE salv_s_ddic_reference.
* Column name
lv_grbew = 'YY_LE_GRBEW'.
* Table name for DDIC reference
ls_grbew_ref-table = 'YMAR_CT_GRBEW'.
* Field in the above mentioned table for DDIC reference
ls_grbew_ref-field = 'YY_LE_GRBEW'.
lt_cols = o_salv->get_columns( ).
ls_col_grbew = lt_cols->get_column(
EXPORTING
columnname = lv_grbew
).
ls_col_grbew->set_ddic_reference(
EXPORTING
value = ls_grbew_ref
).
DATA GR_SALV_FUNC type ref to CL_SALV_FUNCTIONS .
gr_salv_func = o_salv->get_functions( ).
gr_salv_func->set_all( abap_true ).
* Add EDIT function
gr_salv_func->add_function(
name = 'YE_QM_NOTE'
* Optionally add custom text and tooltip
text = lv_edit_text
tooltip = lv_edit_tip
position = if_salv_c_function_position=>right_of_salv_functions ).
* Add SAVE function
gr_salv_func->add_function(
name = 'YE_QM_SAVE'
* Optionally add custom text and tooltip
text = lv_save_text
tooltip = lv_save_tip
position = if_salv_c_function_position=>right_of_salv_functions ).
* Add custom handler method to the table (implementation details follows in the next step)
DATA(lo_events_1) = o_salv->get_event( ).
SET HANDLER alv_1_on_added_function FOR lo_events_1.
* Display the ALV
o_salv->display( ).
METHOD alv_1_on_added_function.
*>------------------------------------------
DATA:ls_api TYPE REF TO if_salv_gui_om_extend_grid_api,
ls_edit TYPE REF TO if_salv_gui_om_edit_restricted,
lv_grbew TYPE lvc_fname,
lv_note TYPE lvc_fname.
* ----------------------
lv_grbew = 'YY_LE_GRBEW'.
lv_note = 'YY_QM_NOTE'.
ls_api = gr_salv_1->extended_grid_api( ).
ls_edit = ls_api->editable_restricted( ).
CASE e_salv_function.
* Note that the cases correspond to the names of the functions we added in step 3)
WHEN 'YE_QM_NOTE'.
* Code for edit enabling goes here - implementation follows in step 5)
WHEN 'YE_QM_SAVE'.
* Code for save goes here - implementation follows in step 6)
WHEN OTHERS.
* Do nothing
ENDCASE.
ENDMETHOD.
IF gv_edit = ''.
gv_edit = 'X'.
ELSE.
gv_edit = ''.
ENDIF.
IF gv_edit = 'X'.
TRY.
* Enable editing
ls_edit->set_attributes_for_columnname(
EXPORTING
columnname = lv_grbew
all_cells_input_enabled = abap_true
).
ls_edit->set_attributes_for_columnname(
EXPORTING
columnname = lv_note
all_cells_input_enabled = abap_true
).
CATCH cx_salv_not_found.
ENDTRY.
ELSE.
TRY.
* Disable editing
ls_edit->set_attributes_for_columnname(
EXPORTING
columnname = lv_grbew
all_cells_input_enabled = abap_false
).
ls_edit->set_attributes_for_columnname(
EXPORTING
columnname = lv_note
all_cells_input_enabled = abap_false
).
CATCH cx_salv_not_found.
ENDTRY.
* When editing is turned off, the user input is checked and data table updated
ls_edit->validate_changed_data(
).
o_salv->refresh( ).
ENDIF.
TRY.
ls_edit->validate_changed_data(
IMPORTING
is_input_data_valid = DATA(s)
).
o_salv->refresh( ).
CATCH cx_salv_not_found.
ENDTRY.
* Input is valid, we can save the data
IF s = 'X'.
* You will need to implement your own save method, based on your own data
save( ).
ENDIF.
And this is it! The removal of inheritance from CL_SALV_TABLE may have broken one method of enabling editing, but fortunately another one was made possible. Once again, this is only possible from release 756 onwards. The previous releases still contain the inheritance and therefore editing can be done by the method described in the method linked at the beginning.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
5 | |
5 | |
3 | |
3 | |
3 | |
2 | |
2 | |
1 | |
1 | |
1 |