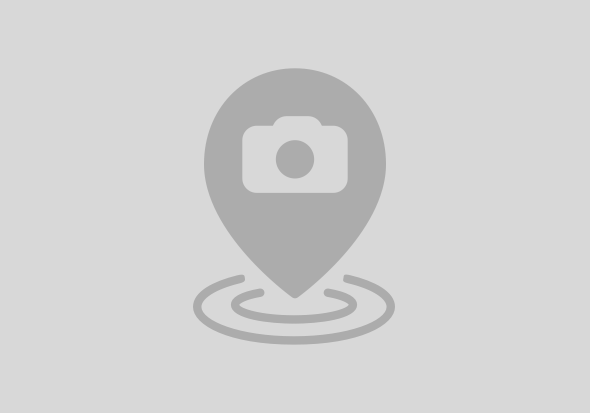
scarr
with a fake-table named zairline_fake
.
CLASS replacement_service_demo DEFINITION FOR TESTING DURATION SHORT
RISK LEVEL HARMLESS.
PRIVATE SECTION.
CLASS-METHODS class_setup.
METHODS read_airlines_from_fake FOR TESTING.
CLASS-METHODS class_teardown.
ENDCLASS.
CLASS replacement_service_demo IMPLEMENTATION.
METHOD class_setup.
DATA: airline_stub TYPE zairline_fake.
cl_osql_replace=>activate_replacement( replacement_table = VALUE #( (
source = 'SCARR' target = 'ZAIRLINE_FAKE' ) ) ).
DELETE FROM zairline_fake.
airline_stub = VALUE #( carrid = 'TG' carrname = 'Thai Airways' currcode = 'THB' ).
INSERT zairline_fake FROM airline_stub.
COMMIT WORK AND WAIT.
ENDMETHOD.
METHOD read_airlines_from_fake.
DATA(expected_airlines) = VALUE scarr_tab( ( carrid = 'TG' carrname = 'Thai Airways' currcode = 'THB' ) ).
SELECT * FROM scarr INTO TABLE @DATA(found_airlines).
cl_abap_unit_assert=>assert_equals( exp = expected_airlines act = found_airlines ).
ENDMETHOD.
METHOD class_teardown.
" stop replacement with empty input parameters
cl_osql_replace=>activate_replacement( ).
ENDMETHOD.
ENDCLASS.
SELECT * FROM scarr
is replaced at runtime by SELECT * FROM zairline_fake
.mseg
or resb
. The next problem was coming soon. It was very difficult to figure out the exact database records, when the OpenSQL replacement should be integraded in an existing test-class. So i started implementing some optimizations.
CLASS exporter DEFINITION ABSTRACT.
PUBLIC SECTION.
METHODS export_from_source
IMPORTING
source_table TYPE tabname
where_restriction TYPE string.
ENDCLASS.
CLASS exporter IMPLEMENTATION.
METHOD export_from_source.
DATA: content TYPE REF TO data.
FIELD-SYMBOLS: <content> TYPE STANDARD TABLE.
CREATE DATA content TYPE STANDARD TABLE OF (source_table).
ASSIGN content->* TO <content>.
SELECT * FROM (source_table) INTO TABLE <content> WHERE (where_restriction).
ENDMETHOD.
ENDCLASS.
CALL TRANSFORMATION id
abap_trans_srcbind_tab
. That makes the serialization of a set of internal tables possible. The result of the identity transformation was exported as binary object with the function module 'WWWDATA_EXPORT'. This binary object could be connected to the transport system.cl_apl_ecatt_tdc_api
the ecatt-test-data containers were more easier to fill.
CLASS import DEFINITION ABSTRACT.
PUBLIC SECTION.
METHODS import
IMPORTING
fake_table TYPE tabname
where_restriction TYPE string
content TYPE STANDARD TABLE.
ENDCLASS.
CLASS importer IMPLEMENTATION.
METHOD import.
DELETE (fake_table) FROM (where_restriction).
INSERT (fake_table) FROM TABLE content.
ENDMETHOD.
ENDCLASS.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
2 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 |