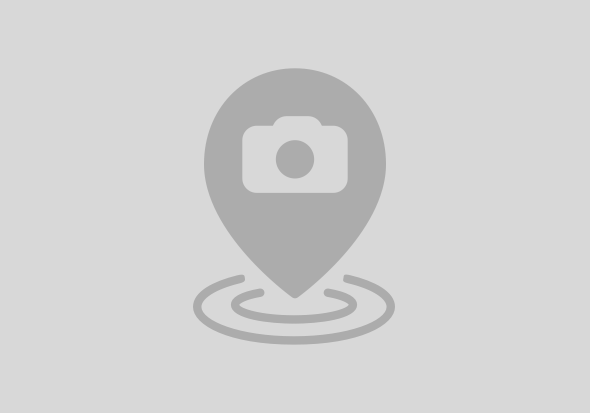
DATA lt_bukrs_rg TYPE RANGE OF bukrs.
APPEND VALUE #( sign = 'I' option = 'EQ' low = '0001' ) TO lt_bukrs_rg.
DO 100000 TIMES.
SELECT FROM t001
FIELDS *
WHERE bukrs IN @lt_bukrs_rg
INTO TABLE @DATA(lt_bukrs).
ENDDO.
CLEAR lt_bukrs_rg.
DO 1000 TIMES.
APPEND VALUE #( sign = 'I' option = 'EQ' low = '0001' ) TO lt_bukrs_rg.
ENDDO.
DO 100000 TIMES.
SELECT FROM t001
FIELDS *
WHERE bukrs IN @lt_bukrs_rg
INTO TABLE @lt_bukrs.
ENDDO.
SORT lt_bukrs_rg.
DELETE ADJACENT DUPLICATES FROM lt_bukrs_rg.
DATA ls_bukrs_rg LIKE LINE OF lt_bukrs_rg.
ls_bukrs_rg-sign = 'I'.
ls_bukrs_rg-option = 'EQ'.
ls_bukrs_rg-low = some value.
READ TABLE lt_bukrs_rg WITH KEY table_line = ls_bukrs_rg BINARY SEARCH TRANSPORTING NO FIELDS.
IF sy-subrc <> 0.
INSERT ls_bukrs_rg INTO lt_bukrs_rg INDEX sy-tabix.
ENDIF.
ls_bukrs_rg = VALUE #( sign = 'I' option = 'EQ' ).
DO 1000 TIMES.
ls_bukrs_rg-low = some value.
COLLECT ls_bukrs_rg INTO lt_bukrs_rg
* Would be nice if you could do COLLECT VALUE #( .. ) but that gives you a syntax * error. You need an explicit work area for the collect.
ENDDO.
TYPES ty_bukrs_rg_tab TYPE RANGE OF bukrs.
TYPES ty_bukrs_rg_ln TYPE LINE OF ty_bukrs_rg_tab.
DATA lth_bukrs_rg TYPE HASHED TABLE OF ty_bukrs_rg_ln
WITH UNIQUE KEY sign option low high.
* The construct above is less typing then:
TYPES BEGIN OF ty_bukrs_rg1.
TYPES sign TYPE ddsign.
TYPES option TYPE ddoption.
TYPES low TYPE bukrs.
TYPES high TYPE bukrs.
TYPES END OF ty_bukrs_rg1.
DATA lth_bukrs_rg1 TYPE HASHED TABLE OF ty_bukrs_rg1
WITH UNIQUE KEY sign option low high.
DO 1000 TIMES.
* The hashed table ensures that we don't have duplicate entries in the ranges table.
INSERT VALUE #( sign = 'I' option = 'EQ' low = '0001' ) INTO TABLE lth_bukrs_rg.
ENDDO.
DATA lth_bukrs_rg TYPE HASHED RANGE OF BUKRS.
* in combination with
INSERT VALUE #( sign = 'I' option = 'EQ' low = '0001' ) INTO TABLE lth_bukrs_rg.
* would be my preferred solution
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
4 | |
3 | |
3 | |
2 | |
2 | |
2 | |
1 | |
1 | |
1 | |
1 |