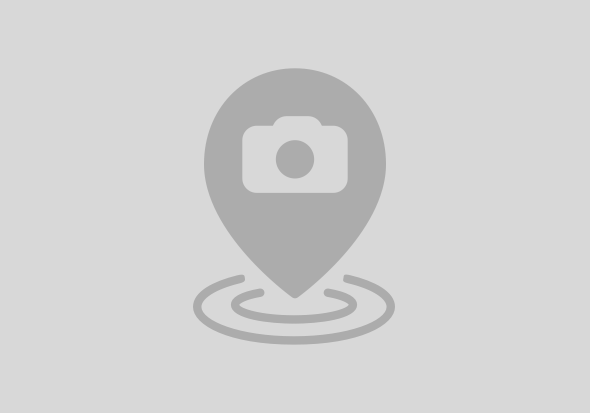
IF mv_given_cat_for_selection IS NOT INITIAL.
" take what is given.
r_result = VALUE #( ( sign = wmegc_sign_inclusive
option = wmegc_option_eq
low = mv_given_cat_for_selection ) ).
ELSE.
" Take cat from first/any one line.
r_result = VALUE #( ( sign = 'I'
option = 'EQ'
low = mt_component_data[ 1 ]-s_v2_items-cat ) ).
ENDIF.
sign = 'I' "no, not so nice
sign = wmegc_sign_inclusive "yes, this I like to use.
IF mv_given_cat_for_selection IS NOT INITIAL.
" take what is given.
rt_range_of_cat = VALUE #( ( sign = wmegc_sign_inclusive
option = wmegc_option_eq
low = mv_given_cat_for_selection ) ).
ELSE.
" Take cat from first/any one line.
rt_range_of_cat = VALUE #( ( sign = 'I'
option = 'EQ'
low = mt_component_data[ 1 ]-s_v2_items-cat ) ).
ENDIF.
DATA ls_range_line LIKE LINE OF rt_range_of_cat.
ls_range_line-sign = wmegc_sign_inclusive.
ls_range_line-option = wmegc_option_eq.
IF mv_given_cat_for_selection IS NOT INITIAL.
" take what is given.
ls_range_line-low = mv_given_cat_for_selection.
ELSE.
" Take cat from first/any one line.
ls_range_line-low = mt_component_data[ 1 ]-s_v2_items-cat.
ENDIF.
INSERT ls_range_line INTO TABLE rt_range_of_cat.
DATA ls_range_line LIKE LINE OF rt_range_of_cat.
IF mv_given_cat_for_selection IS NOT INITIAL.
" take what is given.
ls_range_line-low = mv_given_cat_for_selection.
ELSE.
" Take cat from first/any one line.
ls_range_line-low = mt_component_data[ 1 ]-s_v2_items-cat.
ENDIF.
ls_range_line-sign = wmegc_sign_inclusive.
ls_range_line-option = wmegc_option_eq.
INSERT ls_range_line INTO TABLE rt_range_of_cat.
DATA ls_range_line LIKE LINE OF rt_range_of_cat.
IF mv_given_cat_for_selection IS NOT INITIAL.
ls_range_line-low = mv_given_cat_for_selection.
ELSE.
ls_range_line-low = mt_component_data[ 1 ]-s_v2_items-cat.
ENDIF.
ls_range_line-sign = wmegc_sign_inclusive.
ls_range_line-option = wmegc_option_eq.
INSERT ls_range_line INTO TABLE rt_range_of_cat.
METHOD hu_sel_build_range_for_cat.
DATA ls_range_line LIKE LINE OF rt_range_of_cat.
IF mv_given_cat_for_selection IS NOT INITIAL.
ls_range_line-low = mv_given_cat_for_selection.
ELSE.
ls_range_line-low = mt_component_data[ 1 ]-s_v2_items-cat.
ENDIF.
ls_range_line-sign = wmegc_sign_inclusive.
ls_range_line-option = wmegc_option_eq.
INSERT ls_range_line INTO TABLE rt_range_of_cat.
ENDMETHOD.
METHOD hu_sel_build_range_for_cat.
DATA range_line LIKE LINE OF result_range_of_cat.
IF given_cat_for_selection IS NOT INITIAL.
range_line-low = given_cat_for_selection.
ELSE.
range_line-low = component_data[ 1 ]-s_v2_items-cat.
ENDIF.
range_line-sign = wmegc_sign_inclusive.
range_line-option = wmegc_option_eq.
INSERT range_line INTO TABLE result_range_of_cat.
ENDMETHOD.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
4 | |
3 | |
2 | |
2 | |
2 | |
2 | |
2 | |
1 | |
1 | |
1 |