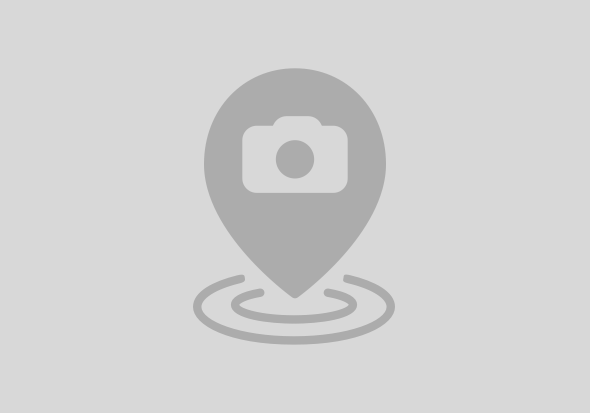
This is a blog series about Application Log, consisting of the following chapters:
In a very normal world, we create log objects to collect messages (from the SYST structure, exceptions, BAPI, or batch input). We then save the log and optionally display it. This means we basically have 4 steps: create, collect, save, and display. In some scenarios, we may have additional requirements such as deleting or inserting messages.
As a developer, I prefer to use a pure standard class that is present in every system and does not contain any specific logic. Does such a class exist? Let's find the answer.
It is possible to reach a list of classes that can be related by searching with CL*LOG* and CL*MESSAGE* patterns in transaction SE24. Eventually something like the following list remains:
Let's include those written in bold text in a drag race¹ and see how functional they are. Others were eliminated because they did not have adequate methods to participate in the race.
⚠️Note that not all of these classes may be available on your system.
First, we will compare some methods of the selected classes. Second, we will write sample code for each of them. Let's see which one crosses the finish line first, 402 meters away. 🏁
CL_BAL_LOGOBJ | CL_PTU_MESSAGE | CL_RECA_MESSAGE_LIST | |
Purpose | Method | Method | Method |
Create log | - CONSTRUCTOR | - CONSTRUCTOR | ℹ️There is a factory class for creating a log. CF_RECA_MESSAGE_LIST=> CREATE |
Add text | - ADD_STATUSTEXT - ADD_ERRORTEXT | - ADD_TEXT - ADD_TIME_STAMP - ADD_EMPTY_LINE | N/A |
Add message | - ADD_MSG | - ADD_MESSAGE - ADD_MESSAGE_SIMPLE - ADD_MESSAGE_COMPLETE | - ADD - ADD_SYMSG |
Add exception | - ADD_EXCEPTION ℹ️Previous messages in the exception chain are not added. | N/A | - ADD_FROM_EXCEPTION ℹ️Previous messages in the exception chain are not added. |
Add BAPI messages | N/A | - ADD_BAPIRET2 - ADD_BAPIRET2_TAB | - ADD_FROM_BAPI |
Add batch input messages | N/A | N/A | N/A |
Insert message | N/A | N/A | - INSERT |
Set level of detail | - DECREMENT_DETLEVEL - INCREMENT_DETLEVEL | Yes, as an input parameter in related methods | - SET_DETAIL_LEVEL |
Cumulate messages | N/A | Yes, as an input parameter in related methods | Yes, as an input parameter in related methods |
Delete message (from memory) | N/A | N/A | - DELETE_MESSAGE |
Delete all messages (from memory) | - REFRESH | - DELETE_MESSAGES | - CLEAR |
Delete log (from DB) | N/A | - DELETE_LOG | N/A |
Get log handle | - GET_HANDLE | - GET_HANDLE | - GET_HANDLE |
Get log number | Yes, as a return parameter when the log saved | Yes, as a return parameter when the log saved | N/A |
Get log header | N/A | - GET_LOG_HEADER | - GET_HEADER |
Get all messages | N/A | - GET_MESSAGES | - GET_LIST - GET_LIST_X - GET_LIST_AS_BAPIRET |
Get statistics | N/A | - GET_MESSAGES | - GET_STATISTICS |
Has messages? (Is empty?) | N/A | - HAS_MESSAGES | - IS_EMPTY - COUNT |
Save log | - SAVE | - SAVE_LOG | - STORE |
Display log | - DISPLAY ✅Display profiles can be used. | - DISPLAY_LOG ⚠️Display profiles can not be used. | ℹ️There is no method to display the log, but function RECA_GUI_MSGLIST_POPUP can be used. |
N/A: Not available
Now let's take a look at some simple code examples of how to use these classes.
📄ABAP code
TRY. " Create the log DATA(appl_log) = NEW cl_bal_logobj( i_log_object = 'APPL_LOG' i_default_subobject = 'OTHERS' i_extnumber = 'CL_BAL_LOGOBJ' ). " Add a message MESSAGE s361(00) INTO DATA(msgtext). appl_log->add_msg( i_probclass = if_bal_logger=>c_probclass_none ). " Add an exception TRY. RAISE EXCEPTION TYPE cx_demo_constructor. CATCH cx_demo_constructor INTO DATA(exception). appl_log->add_exception( exception ). ENDTRY. " Save the log appl_log->save( IMPORTING et_lognumbers = DATA(log_numbers) ). " Display the log appl_log->display( ). CATCH cx_bal_exception. ENDTRY. "TRY . " cl_demo_output=>write( |CL_BAL_LOGOBJ log number: { log_numbers[ 1 ]-lognumber }| ). " cl_demo_output=>write( |CL_BAL_LOGOBJ log handle: { log_numbers[ 1 ]-log_handle }| ). " cl_demo_output=>display( ). " CATCH cx_root. "ENDTRY.
🖥️ Output
![]() |
💭Comments
This class is included in SAP standard. (Package: SZAL, Application Component: BC-SRV-BAL). Although it does not contain methods for adding BAPI and BDC messages, it can be used for basic needs. Note that there is no method that returns the collected messages. Therefore, this class can be used to collect messages and to save and display logs.
☢️Important note This class also has a method called ADD_DEBUG_MESSAGE which can be used to add a message with the call stack. If this method is used with the parameter I_DETLEVEL and the log is displayed with the display profile "hierarchy by message detail level", a runtime error CONVT_NO_NUMBER occurs. This is because the following line in CL_BAL_LOG_BASE->IF_BAL_LOGGER~ADD_DEBUG_MESSAGE has an incorrect assignment: l_s_msg-detlevel = if_bal_logger~mv_loghandle. The runtime error occurs in the DETLEVEL_FILL macro in LSBAL_DISPLAY_BASEF05. (Line 344). Therefore, the ADD_DEBUG_MSG method should not be used when messages are to be displayed by detail level. An example code to reproduce the error: DATA display_profile TYPE bal_s_prof. CALL FUNCTION 'BAL_DSP_PROFILE_DETLEVEL_GET' IMPORTING e_s_display_profile = display_profile. TRY. DATA(log) = NEW cl_bal_logobj( ). log->add_debug_msg( i_detlevel = '1' ). log->display( i_disp_profile = display_profile ). CATCH cx_bal_exception . ENDTRY. |
📄ABAP code
" Create the log DATA appl_log TYPE REF TO cl_ptu_message. DATA(log_header) = VALUE bal_s_log( object = 'APPL_LOG' subobject = 'OTHERS' extnumber = 'CL_PTU_MESSAGE' ). CREATE OBJECT appl_log EXPORTING is_log = log_header EXCEPTIONS OTHERS = 3. CHECK appl_log IS BOUND. " Add a message MESSAGE s361(00) INTO DATA(msgtext). appl_log->add_message_simple( EXPORTING iv_level = CONV #( if_bal_logger=>c_probclass_none ) ). " Add BAPI messages DATA bapiret2_tab TYPE bapiret2_tab. CALL FUNCTION 'BAPI_FLIGHT_GETDETAIL' EXPORTING airlineid = VALUE bapisflkey-airlineid( ) connectionid = VALUE bapisflkey-connectid( ) flightdate = VALUE bapisflkey-flightdate( ) TABLES return = bapiret2_tab. appl_log->add_bapiret2_tab( EXPORTING it_bapiret2 = bapiret2_tab ). " Save the log appl_log->save_log( IMPORTING es_new_lognumber = DATA(log_number) EXCEPTIONS OTHERS = 2 ). " Display the log appl_log->display_log( EXPORTING iv_as_popup = abap_true iv_use_grid = abap_true EXCEPTIONS OTHERS = 2 ). "cl_demo_output=>write( |CL_PTU_MESSAGE log number: { log_number-lognumber }| ). "cl_demo_output=>write( |CL_PTU_MESSAGE log handle: { log_number-log_handle }| ). "cl_demo_output=>display( ).
🖥️ Output
![]() |
💭Comments
Although it is possible to collect BAPI return messages with this class, it does not include a method for collecting BDC messages. There is also no method for collecting exception messages. The inability to use display profiles is another disadvantage.
📄ABAP code
" Create the log DATA(msg_list) = cf_reca_message_list=>create( id_object = 'APPL_LOG' id_subobject = 'OTHERS' id_extnumber = 'CL_RECA_MESSAGE_LIST' ). CHECK msg_list IS BOUND. DATA(log_header) = msg_list->get_header( ). log_header-params-altext = 'Appl. log: Standard text'. msg_list->change_header( EXPORTING is_msg_header = log_header EXCEPTIONS OTHERS = 2 ). " Add a message MESSAGE s361(00) INTO DATA(msgtext). msg_list->add_symsg( EXPORTING id_probclass = if_bal_logger=>c_probclass_none ). " Add BAPI messages DATA bapiret2_tab TYPE bapiret2_tab. CALL FUNCTION 'BAPI_FLIGHT_GETDETAIL' EXPORTING airlineid = VALUE bapisflkey-airlineid( ) connectionid = VALUE bapisflkey-connectid( ) flightdate = VALUE bapisflkey-flightdate( ) TABLES return = bapiret2_tab. msg_list->add_from_bapi( EXPORTING it_bapiret = bapiret2_tab ). " Add an exception TRY. RAISE EXCEPTION TYPE cx_demo_constructor. CATCH cx_demo_constructor INTO DATA(exception). msg_list->add_from_exception( io_exception = exception ). ENDTRY. " Save the log msg_list->store( EXPORTING if_in_update_task = abap_false EXCEPTIONS OTHERS = 2 ). " Display the log CALL FUNCTION 'RECA_GUI_MSGLIST_POPUP' EXPORTING io_msglist = msg_list. "cl_demo_output=>write( |CL_RECA_MESSAGE_LIST log number: Who knows? | ). "cl_demo_output=>write( |CL_RECA_MESSAGE_LIST log handle: { msg_list->get_handle( ) }| ). "cl_demo_output=>display( ).
🖥️ Output
![]() |
💭Comments
This is the richest class in terms of method in the race. Strangely, it has no method for adding free text. Like other classes, it has no method for collecting BDC messages. The good thing is that there is a method for inserting messages. An original feature is the possibility to display the log in a resizable window. (The next chapter is about it)
⚠️This class may not be available in every system.
As you can see, each class has different methods. One has a method for collecting BAPI return messages, while the other has a method for collecting exception messages. One does not have a method to add custom text, while the other includes a method to add a message with a date/time stamp. However, not all three classes have a method for collecting batch input messages.
So who is the winner? Although none of the classes in the race really ticked all the boxes, the winner seems to be CL_RECA_MESSAGE_LIST.
Before we take a custom class to the next race, let's make a pit stop here and take a look at a nice feature of the winner: Displaying an Application Log in a Resizable Window.
See you in the next chapter.
More Resources
|
Endnotes
|
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
4 | |
3 | |
3 | |
2 | |
2 | |
2 | |
1 | |
1 | |
1 | |
1 |