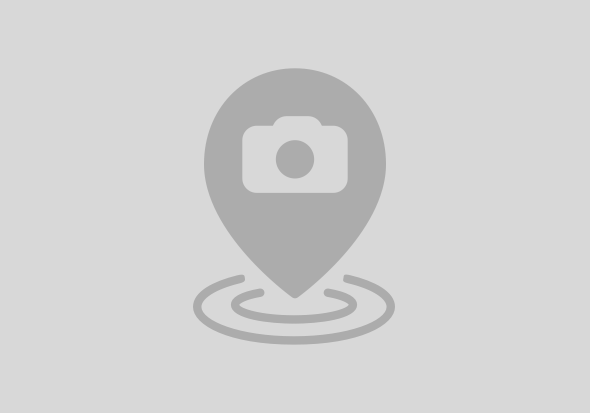
SAP Sales and Service Cloud Version 2 provides KPI Cards inside Account Details, which are called "Key Metrics". Key Metrics provide quick information about the transactional documents of the account displayed.
Standard Key Metrics
Besides SAP Delivered KPI Cards, you have the option to create your own Key Metrics by fetching the data from outside, into SAP's pre-delivered templates. In this example we will be using Kyma Serverless Functions and API Rules to create our own Customer Key Metrics. The example will cover both Sales and Service Cloud Version 2 configuration and the Kyma Configuration.
Before we start, I'd like to express that Custom Key Metrics can work with any language, as long as you can parse and return JSON a payload. You may have an analytics application on your hand, you may have Integration Suite knowledge and they would work just as well. The reason I went with Kyma is because it handles all the DevOps for you, automatically scales and provides secure ways of exposing your functions.
Before we have can start our example, please make sure that you are fulfilling the prerequisites below.
In our example we will create a custom key metric that will show how many leads are created from which sources for this account. Picture below shows the final result in an account page.
To explain how the Custom Key Metrics works:
//Example Pie chart JSON Object that needs to be returned in the payload
{
"total": "{calculated results}",
"data": [
{
"name": "{description}",
"y": "{calculated result}"
},
{
"name": "{description}",
"y": "{calculated result}"
}
//and so on...
]
}
Before going to Sales and Service Cloud Version 2, first we need to create our function and endpoint on Kyma, as we need to provide this information in the System.
Open your Kyma Environment, go to your desired namespace.
Go to Workloads -> Functions -> + Create Function
Note: In our example "lodash" library is not used and does not need to be added. But in general, it is a useful library for object manipulation.
We now have a serverless function available. The next step is to add some environment variables to make this function easily maintainable across different namespaces (like dev-test-prod) without changing the source code.
We are going to add the following environment variables for our example:
Secrets provide a secure and easy way to access your credentials in Kyma Environment. You can declare them as variables in your code and manage them centrally, without modifying your source code.
In Kyma, go to Configuration -> Secrets -> Create Secret
You now have a secret that you can use in any function that you create.
Go back to your function, click on edit and on the environment variable section, add the following values as shown below.
YAML:
env:
- name: CNS_SECRET
valueFrom:
secretKeyRef:
key: password
name: cns-key-insights-user
- name: CNS_USERNAME
valueFrom:
secretKeyRef:
key: username
name: cns-key-insights-user
- name: CNS_URL
value: https://YOURCNSTENANT/sap/c4c/api/v1
Now we can fetch these environment variables in our code:
//environment variables
const cnsSecret = process.env.CNS_SECRET;
const cnsUsername = process.env.CNS_USERNAME;
const cnsUrl = process.env.CNS_URL;
With API Rules, we can create an endpoint for our functions - or our services in general. We are able to define different paths, routed to different functions with different security options for each path.
Go to Discovery and Network -> API Rules -> Create API Rule
YAML:
- accessStrategies:
- config:
jwks_urls:
- https://youriastenant.accounts.ondemand.com/oauth2/certs
trusted_issuers:
- https://youriastenant.accounts.ondemand.com
handler: jwt
methods:
- POST
Now that we have everything ready, we can finalize our function. Go back to the function you have created.
Click edit and add the source code below:
const axios = require('axios');
// Load environment variables
const cnsSecret = process.env.CNS_SECRET;
const cnsUsername = process.env.CNS_USERNAME;
const cnsUrl = process.env.CNS_URL;
module.exports = {
main: async function (event, context) {
try {
console.log('Event Received:', JSON.stringify(event.data));
// Extract businessPartnerId from event data
const businessPartnerId = event.data.businessPartnerId;
// Define parameters object
const params = {
'$filter': `account.id eq '${businessPartnerId}'`,
'$select': 'name,id,sourceDescription'
};
// Perform GET request with basic authentication and parameters
const leadResponse = await axios.get(`${cnsUrl}/lead-service/leads`, {
auth: {
username: cnsUsername,
password: cnsSecret,
},
params,
});
// Extract leads data
const leads = leadResponse.data.value;
console.log("Fetched Leads: "+JSON.stringify(leads))
// Calculate total count of leads
const total = leads.length;
console.log("Count of Leads: "+JSON.stringify(total))
// Group leads by sourceDescription and count occurrences
const groupedData = leads.reduce((acc, lead) => {
const name = lead.sourceDescription;
acc[name] = (acc[name] || 0) + 1;
return acc;
}, {});
console.log("groupedData: "+JSON.stringify(groupedData));
// Convert object to array with name and count
const data = Object.entries(groupedData).map(([name, count]) => ({ name, y: count }));
// Construct response payload
const payload = {
total,
data,
};
console.log(JSON.stringify(payload));
return payload;
} catch (error) {
console.error(error);
return { error: error.message };
}
},
};
As mentioned earlier, this code has been generated by AI, and slightly modified by hand. As long as you give a strong prompt, AI can handle these simple scripts really well.
Here's my example prompt:
/*
I need you to write me a nodejs script, which will be used in Kyma environment, you can refer to https://github.com/kyma-project. I have the following config yaml, with some environment variables. I need you to save these environments variables as a constant in the code to be used. You may use axios and lodash libraries.
env:
- name: CNS_SECRET
valueFrom:
secretKeyRef:
name: cns-key-insights-user
key: password
- name: CNS_USERNAME
valueFrom:
secretKeyRef:
name: cns-key-insights-user
key: username
- name: CNS_URL
value: https://host/sap/c4c/api/v1
schemaVersion: v0
Code will be triggered via an HTTP Request Event. When the event gets triggered, log the event headers and data separately.
example event data: {"businessPartnerId":"11edee65-fccb-955e-afdb-817c5e020a00","billingDocumentId":null,"top":0,"sortBy":null,"idType":null,"language":"en"}
Make a GET request to /lead-service/leads path. for URL, use the constant you have created for CNS_URL environment variable.
Use the query parameter: $filter=account.id eq '{businessPartnerId from event}'
Count the total of records returned. Add it into "total" key of the payload. Count the sourceDescription, group the sourceDescription, add it into "data" array of the response payload. "name" should be the grouped sourceDescription, y should is the count of the grouped sourceDescription.
Return the following payload in the end.
{
"total": "{count of leads}",
"data": [
{
"name": "{sourceDescription1}",
"y": "{count of sourceDescription1}"
},
{
"name": "{sourceDescription2}",
"y": "{count of sourceDescription2}"
}
]
}
*\
Now that we have our code up and running, it is time to configure the Sales and Service Cloud Version 2 side.
Of course, always treat these codes cautiously as AI tends to make a lot of mistakes. Also, make sure to test different cases and modify your code accordingly to handle those cases.
Once you have everything ready on Kyma side, configuration on CNS side (Cloud Native Stack - a.k.a. Sales and Service Cloud Version 2) will come pretty easily.
If you would like to use a specific user for Key Insights (which I'd recommend) you need to create a new Communication System.
Go to Settings -> Integration -> Communication Systems and click on plus (+) icon.
Inbound settings will create a technical user that you will use to fetch data from the APIs of your CNS Tenant. Set up a password and click on Save and Activate. Your technical user with the same name will be created in the background.
Click edit on your communication system and open the Outbound settings.
Outbound setting is where you fill in the receiver system's credentials and link.
Host Name: Add the link that you created in the Kyma API Rules. Do not add paths (/example/path).
Authentication Method: For the authentication, if you have followed the blog and used JWT, then use your IAS OAuth Client Credentials as shown above. To create your own IAS OAuth Keys, click here.
Go to Settings -> Customer Insights -> Custom Key Metrics and click on plus (+) icon.
Key Metric Name: Administrative name of the key metric
Key Metric Title: The name that you will see in Accounts Key Metrics section.
Chart Type: Choose Pie, as we have used that payload type in the function.
API Path: Use the path that you have defined for your function in the API Rules.
Communication System: Select the system that you have created earlier.
Navigation URL: If you want to launch a specific link, you can place it here.
Click Save. Now you have configured your custom key metric!
To test your Key Metrics, go to any Accounts that have leads. On the Key Metrics section, custom key metrics will be on the last cards.
You can trace the network logs by searching "key-metrics".
In the Kyma logs, you can trace your function real time and see any errors that come up.
Now you have a functioning Key Metric at your service! Good thing is, first one is actually the most time consuming but for the future, you can re-use the first one as a template for the other key metrics that you can create.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
1 | |
1 | |
1 | |
1 | |
1 |