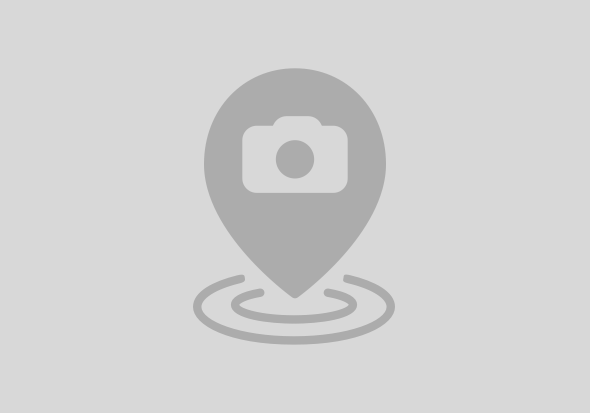
namespace sap.dealmakers;
entity BidOutcomes {
key bidId : UUID;
reqUnderstoodRate : Integer;
fitmentVsRequiredFeatureRatio : Integer;
identifiedServicesAndDeliveryOptions : String;
solutionValidated : String;
riskRedressalDone : String;
clarificationAddressed : String;
pocRequested : String;
bidChecklistFollowed : String;
referencesPastProposals : String;
isCompetitorProductExpiring : String;
dealSize : String;
discountSlabOffered : Integer;
cLevelExecInMeeting : String;
}
using sap.dealmakers from '../db/hdb';
service DealsService {
entity BidOutcomes as projection on dealmakers.BidOutcomes;
}
/*eslint no-console: 0*/
// Get all the libraries required for processing
const axios = require('axios');
var fs = require('fs');
var url = require('url');
var http = require('http');
var AdmZip = require('adm-zip');
var request = require('request');
// Pass your survey id
var surveyid = 'SV_eDIL1bRzKlebXXX'
// Pass your API token from your qualtrics account
var headers = {
'X-API-TOKEN': 'dBCclMcOWOYCMgquJtcsplJIw59W6jy2lcjXXXXX',
'Content-Type': 'application/json'
};
// Declare the data format for processing
var dataString = '{"format": "json" }';
// Prepare the URL for the XM platform
var options = {
url: 'https://xxxxx.qualtrics.com/API/v3/surveys/' + surveyid + '/export-responses',
method: 'POST',
headers: headers,
body: dataString
};
// Trigger call to fetch the data from Qualtrics platform
function callback(error, response, body) {
if (!error && response.statusCode == 200) {
var options2 = {
url: 'https://xxxxx.qualtrics.com/API/v3/surveys/' + surveyid + '/export-responses/' + JSON.parse(response.body).result.progressId,
headers: headers
};
var intervalComplete = false;
function callback2(error, response, body2) {
if (!error && !intervalComplete && response.statusCode === 200 && JSON.parse(response.body).result.status === "complete") {
clearInterval(intervalId);
intervalComplete = true;
var options3 = {
url: 'https://xxxxx.qualtrics.com/API/v3/surveys/' + surveyid + '/export-responses/' + JSON.parse(response.body).result.fileId +
'/file',
headers: headers,
encoding: null
};
function callback3(error, response, body) {
if (!error && response.statusCode == 200) {
// Qualtrics exports the survey response in ZIP format
if (response.headers['content-type'] == 'application/zip') {
var zip = new AdmZip(body);
var zipEntries = zip.getEntries();
var json = JSON.parse(zip.readAsText(zipEntries[0]));
var oData = {};
//Unzip the response and parse the results in format which is mapped with the DB table structure
json.responses.forEach(resp => {
console.log("--- Response is ----");
oData = {
"dealStatus": resp.labels.QID11,
"cLevelExecInMeeting": resp.labels.QID1,
"numberOfMeetings": resp.values.QID13_TEXT,
"competitorsInvolvedInBidding": resp.labels.QID3 ,
"demoRequested": resp.labels.QID6,
"demoDelivered": resp.labels.QID14 ,
"cLevelExecInDemo": resp.values.QID15,
"demoFeedbackRating": resp.values.QID16_1,
"wasFollowupAfterDemoRequested": resp.labels.QID8,
"discountSlabOffered": resp.values.QID17_TEXT,
"sapSolutionsOffered": resp.values.QID18_TEXT,
"reqUnderstoodRate": resp.values.QID20_1,
"fitmentVsRequiredFeatureRatio": resp.values.QID22_TEXT,
"identifiedServicesAndDeliveryOptions": resp.labels.QID23,
"solutionValidated": resp.labels.QID24,
"riskRedressalDone": resp.labels.QID25,
"clarificationAddressed": resp.labels.QID26,
"bidChecklistFollowed": resp.labels.QID29,
"isCompetitorProductExpiring": resp.labels.QID30,
"referencesPastProposals": resp.labels.QID31
};
console.log("--- POSTING Odata ----", oData);
// Prepare fully qualified URL for the ODATA service which will hit the table and write the data in the HANA container
axios.post('https://xxxxx.cfapps.eu10.hana.ondemand.com/deals/BidOutcomes', oData)
.then((res) => {
console.log(`statusCode: ${res.statusCode}`);
})
.catch((error) => {
console.error(error);
});
});
console.log('CALLBACK4');
}
}
}
// Download the survey responses .zip
request(options3, callback3);
}
}
var intervalId = setInterval(function () {
// Trigger to get progress update
console.log("triggering progress update call and waiting...");
request(options2, callback2);
}, 500);
}
else{
response.write("Failed!!");
}
}
// Create Export and get ProgressID
request(options, callback);
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
10 | |
8 | |
4 | |
2 | |
1 | |
1 | |
1 | |
1 | |
1 |