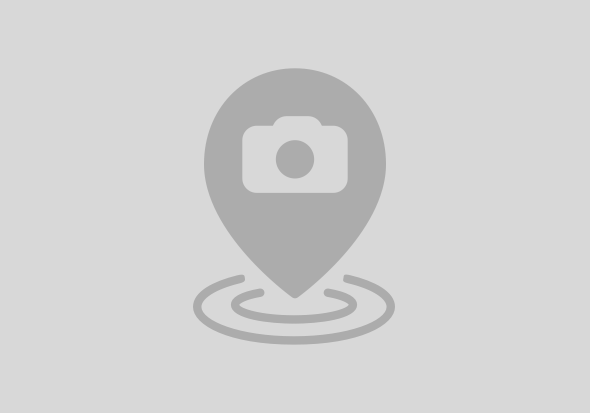
Here, few things to notice.
CLASS zdmo_ads_assistance DEFINITION
PUBLIC
FINAL
CREATE PUBLIC .
PUBLIC SECTION.
TYPES: BEGIN OF ty_doc,
docnum(10) TYPE c,
docchild(10) TYPE c,
END OF ty_doc,
ts_docs TYPE ty_doc,
BEGIN OF struct,
xdp_template TYPE string,
xml_data TYPE string,
form_type TYPE string,
form_locale TYPE string,
tagged_pdf TYPE string,
embed_font TYPE string,
END OF struct,
BEGIN OF ty_header,
DocumentNumber(10) TYPE c,
DocumentStatus(12) TYPE c,
ShortDescription(40) TYPE c,
ChangeResponsible(40) TYPE c,
END OF ty_header,
BEGIN OF ty_footer,
PrintedOn(10) TYPE c,
GeneratedBy(40) TYPE c,
END OF ty_footer,
BEGIN OF ty_print_data,
header TYPE TY_header,
footer TYPE ty_footer,
END OF ty_print_data.
METHODS: generate_ads_forms IMPORTING iv_documents TYPE ts_docs
RAISING cx_web_http_client_error.
PROTECTED SECTION.
PRIVATE SECTION.
ENDCLASS.
CLASS zdmo_ads_assistance IMPLEMENTATION.
METHOD generate_ads_forms.
FIELD-SYMBOLS: <fs_val_ref> TYPE data.
DATA: ls_print_data TYPE ty_print_data,
lv_file_content TYPE string,
lo_val_ref TYPE REF TO data.
FIELD-SYMBOLS: <fs_pdf_data> TYPE data,
<fs_pdf_encoded> TYPE string,
<fs_file_name> TYPE data.
CONSTANTS: lc_success TYPE i VALUE 200.
" Sample Data for the form
ls_print_data-footer-generatedby = 'Shin-Chan N'.
ls_print_data-footer-printedon = '01/12/2022'.
ls_print_data-header-changeresponsible = 'Buri Buri Zaemon'.
ls_print_data-header-documentnumber = '0030002084'.
ls_print_data-header-documentstatus = 'Closed'.
ls_print_data-header-shortdescription = 'Adventures of Buri Buri Zaemon'.
TRY.
* First Let us create the destination of the API
DATA(lo_destination_instance) = cl_http_destination_provider=>create_by_cloud_destination(
i_name = 'ADOBE_FORMS_DEST_CF'
i_service_instance_name = 'ZDMO_COM_0503'
i_authn_mode = if_a4c_cp_service=>service_specific
).
* Second client is created
DATA(lo_http_client) = cl_web_http_client_manager=>create_by_http_destination( i_destination = lo_destination_instance ).
* Then create the request instance from the Client
DATA(lo_request_obj) = lo_http_client->get_http_request( ).
* Let us now create header of the request
DATA lv_header_fields TYPE if_web_http_request=>name_value_pairs.
lv_header_fields = VALUE #(
( name = 'Accept' value = 'application/json, text/plain, */*' ) ##NO_TEXT
( name = 'Content-Type' value = 'application/json;charset=utf-8' ) ).
lo_request_obj->set_header_fields( i_fields = lv_header_fields ).
* Set the api URI for the request
lo_request_obj->set_query( query = 'templateSource=storageName' ).
lo_request_obj->set_uri_path( i_uri_path = '/v1/adsRender/pdf' ).
** Lets create the xml data from the sample data we have created at the beginning.
DATA(lo_xml_conv) = cl_sxml_string_writer=>create( type = if_sxml=>co_xt_xml10 ).
CALL TRANSFORMATION zdmo_ads_form SOURCE root = ls_print_data RESULT XML lo_xml_conv.
DATA(lv_output_xml) = lo_xml_conv->get_output( ).
DATA(ls_data_xml) = cl_web_http_utility=>encode_x_base64( lv_output_xml ).
** Lets create the form template
DATA(ls_form_structure) = VALUE struct( xdp_template = 'ZDMO_ADS_FORM/ADS_DOCUMENT_TEMPLATE'
xml_data = ls_data_xml
form_type = 'dynamicInteractive'
form_locale = 'en_US'
tagged_pdf = '0'
embed_font = '0' ).
DATA(lv_formmated_json) = /ui2/cl_json=>serialize( data = ls_form_structure compress = abap_true pretty_name = /ui2/cl_json=>pretty_mode-camel_case ).
lo_request_obj->set_text( EXPORTING i_text = lv_formmated_json ).
** Execute the request and get the response object
DATA(lo_response_obj) = lo_http_client->execute( i_method = if_web_http_client=>post ).
IF lo_response_obj IS BOUND AND lo_response_obj->get_status( )-code EQ lc_success.
** Success
ENDIF.
CATCH cx_root INTO DATA(lv_error).
** Report errors here
ENDTRY.
ENDMETHOD.
ENDCLASS.
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
6 | |
5 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 | |
3 | |
2 |