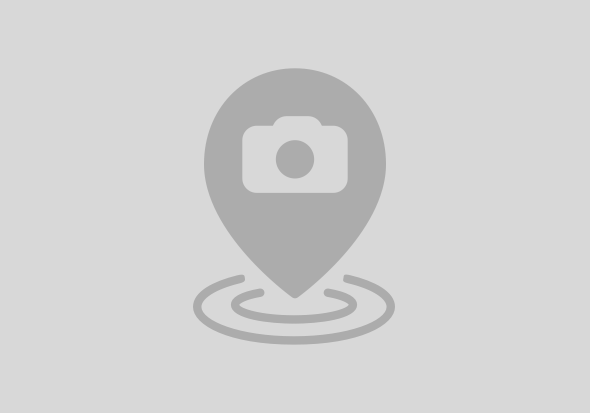
Hi all,
Many of us are leveraging SAP CAP (Node.js with TypeScript) for our projects.
While CAP provides an abstract mechanism to derive RESTful oData services given a CDS data model there's always room for more efficiency and improvement in the implementing communication between business logic, service, and persistence layer.
It shall be noted at this point, that improvements have been attempted with regard to the service layer communication using cds-routing-handler However, this framework does not appear to be maintained, especially for newer versions of CAP.
1. CDS-TS-Dispatcher: Simplifying SAP CAP TypeScript Development
2. CDS-TS-Repository: Simplify SAP CAP Entity persistance with BaseRepository
3. SAP CAP: Controller - Service - Repository design pattern
Crafted by developers for developers by ABS & DxFrontier team
In this blog, we will concentrate on the CDS-TS-Repository.
Before we dive in, you should have a basic understanding of SAP CAP NodeJS and TypeScript. If you need a refresher, here are some helpful resources Official SAP CAP TypeScript
The goal of CDS-TS-Repository - BaseRepository is to significantly reduce the boilerplate code required to implement data access layers for persistence entities by providing out-of-the-box actions on the database.
Now, let's get started with CDS-TS-Repository. We'll walk you through the steps to integrate it into your SAP CAP TypeScript project, providing code snippets and configuration examples for a smooth onboarding.
1: Install cds-ts-repository NPM package.
npm install @dxfrontier/cds-ts-repository
2. Generate type entities using cds-typer
npx -js/cds-typer "*" --outputDirectory ./srv/util/types/entities
The cds-typer offers a way to derive TypeScript definitions from a CDS model to give you enhanced code completion and a certain degree of type safety when implementing services.
3: MyRepository class
Start by creating a MyRepository class, which will extend the BaseRepository to handle operations for your entity
import { BaseRepository } from '@dxfrontier/cds-ts-repository';
import { MyEntity } from 'YOUR_CDS_TYPER_ENTITIES_LOCATION';
class MyRepository extends BaseRepository<MyEntity> {
constructor() {
super(MyEntity) // a CDS Typer entity type
}
aMethod() {
// BaseRepository predefined methods using 'MyEntity' entity
// All methods parameters will allow only keys/values of type MyEntity
const result1 = await this.create(...)
const result2 = await this.createMany(...)
const result5 = await this.getAll()
const result6 = await this.getAllAndLimit(...)
const result7 = await this.find(...)
const result8 = await this.findOne(...)
const result9 = await this.delete(...)
const result10 = await this.update(...)
const result11 = await this.updateLocaleTexts(...)
const result12 = await this.exists(...)
const result13 = await this.count()
const results14 = await this.getLocaleTexts()
const results15 = await this.builder().find(...).orderAsc([...]).getExpand(['...']).execute()
// ...
}
}
Let's see how CDS-TS-Repository simplifies common tasks.
import { BaseRepository } from '@dxfrontier/cds-ts-repository';
import { MyEntity } from 'YOUR_CDS_TYPER_ENTITIES_LOCATION';
class MyRepository extends BaseRepository<MyEntity> {
constructor() {
super(MyEntity);
}
public async aMethod() {
const created = await this.create({
name : 'a new entry',
description : 'description'
})
// update
const keys = { ID : '34' }
const fieldsToUpdate = { name : 'updated entry with a new name' }
// updated will return boolean (true if updated, false otherwise)
const updated = await this.update(keys, fieldsToUpdate)
// exists will return boolean (true if exists, false otherwise)
const exists = await this.exists({ID : '84c67833-a9cb-450c-ae02-a32c3a7a6f6b'})
// results will contain an Array of your entity object
const results = await this.getAll();
// delete will contain true if delete operation is successfull, and false otherwise
const deleted1 = await this.delete({ name: 'Customer' });
const deleted2 = await this.delete({ ID: '2f12d711-b09e-4b57-b035-2cbd0a02ba19' });
// count the number of items
const itemsLength = await this.count();
}
BaseRepositoryDraft repository provides a clear separation of methods for working with active entities and draft instances.
import { BaseRepository, BaseRepositoryDraft, Mixin} from '@dxfrontier/cds-ts-repository'
import { MyEntity } from 'YOUR_CDS_TYPER_ENTITIES_LOCATION'
class MyRepository extends Mixin(BaseRepository<MyEntity>, BaseRepositoryDraft<MyEntity>) {
constructor() {
super(MyEntity)
}
// ... define custom CDS-QL actions if BaseRepository ones are not satisfying your needs !
}
Use BaseRepository methods when dealing with active entity instances.
Use BaseRepositoryDraft methods when working with draft entity instances.
Mixin BaseRepository and BaseRepositoryDraft
For more info about CDS-TS-Repository visit the following GitHub
In conclusion, CDS-TS-Dispatcher combined with CDS-TS-Repository is a powerful tool that can speed up your SAP CAP TypeScript projects by eliminating repetitive code and being a better fit for common team architecture setups.
Whether you're starting a new project or enhancing an existing one, integrating CDS-TS-Repository is a significant step towards a more efficient and productive development journey.
Find an example of usage of the CDS-TS-Samples GitHub
Like
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
7 | |
5 | |
5 | |
5 | |
4 | |
4 | |
3 | |
3 | |
3 | |
3 |