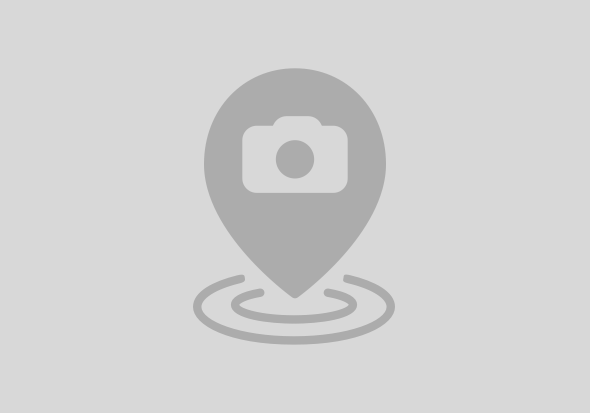
managed
early numbering. It is managed
because RAP runtime engine generates and assigns values to primary key fields.unmanaged
early numbering. It is unmanaged
because:FOR NUMBERING
Tables and Foreign Key Relationships
ZDH_MngdDocumentHeader
is the root entity:CDS Model
Initial Behavior Definition as generate
early numbering
for each entity that needs to implement this. Notice the highlighted lines in the BDEF below:Behavior Definition Updated
Field Mapping
Initial Behavior Implementation Class
early numbering
defined, there is a method generated. Notice the FOR NUMBERING
in the method definition.MODIFY ENTITIES OF... CREATE...
or MODIFY ENTITIES OF.... CREATE BY...
for an entity, respective FOR NUMBERING
method is called.ZCL_DH_MNGD_DOCUMENT_HANDLER
which has 3 methods:GET_NEXT_DOC_NUMBER
-> returns next available document number. How it is done is not so importantGET_NEXT_ITEM_NUMBER
-> returns next item number for a given documentGET_NEXT_ACCOUNT_NUMBER
-> returns next account number for a given document itemEARLYNUMBERING_
methods of BIL class, for each created instance (%CID), generate a new number and then fill “mapped” parameter.These methods are called before any determination ... on modify { create; }
are called
EARLYNUMBERING_CREATE
method, notice that we are filling MAPPED-HEADER
by calling GET_NEXT_DOC_NUMBER()
method: METHOD earlynumbering_create.
DATA(lo_doc_handler) = zcl_dh_mngd_document_handler=>get_instance( ).
LOOP AT entities ASSIGNING FIELD-SYMBOL(<ls_entity>).
INSERT VALUE #( %cid = <ls_entity>-%cid
DocumentNumber = lo_doc_handler->get_next_doc_number( ) ) INTO TABLE mapped-header.
ENDLOOP.
ENDMETHOD.
EARLYNUMBERING_CBA_ITEM
method, we are filling MAPPED-ITEM
by calling GET_NEXT_ITEM_NUMBER()
method. Notice that we are passing the DocumentNumber
to this method which is available in the importing parameter entities
:METHOD earlynumbering_cba_Item.
DATA(lo_doc_handler) = zcl_dh_mngd_document_handler=>get_instance( ).
LOOP AT entities ASSIGNING FIELD-SYMBOL(<ls_entity>).
LOOP AT <ls_entity>-%target ASSIGNING FIELD-SYMBOL(<ls_item_create>).
INSERT VALUE #( %cid = <ls_item_create>-%cid
DocumentNumber = <ls_entity>-DocumentNumber
ItemNumber = lo_doc_handler->get_next_item_number( iv_doc_number = <ls_entity>-DocumentNumber ) ) INTO TABLE mapped-item.
ENDLOOP.
ENDLOOP.
ENDMETHOD.
EARLYNUMBERING_CBA_ACCOUNT
as well. METHOD earlynumbering_cba_Account.
DATA(lo_doc_handler) = zcl_dh_mngd_document_handler=>get_instance( ).
LOOP AT entities ASSIGNING FIELD-SYMBOL(<ls_entity>).
LOOP AT <ls_entity>-%target ASSIGNING FIELD-SYMBOL(<ls_account_create>).
INSERT VALUE #( %cid = <ls_account_create>-%cid
DocumentNumber = <ls_entity>-DocumentNumber
ItemNumber = <ls_entity>-ItemNumber
AccountNumber = lo_doc_handler->get_next_account_number( iv_doc_number = <ls_entity>-DocumentNumber
iv_item_number = <ls_entity>-ItemNumber ) ) INTO TABLE mapped-account.
ENDLOOP.
ENDLOOP.
ENDMETHOD.
Defaults
to fill administrative fields such as CreatedBy, CreatedOn
. Complete listing of BIL class as well as ZCL_DH_MNGD_DOCUMENT_HANDLER
is available on the GitHub repo.MODIFY ENTITIES OF ZDH_MngdDocumentHeader
ENTITY Header
CREATE SET FIELDS WITH VALUE #( ( %cid = 'doc1'
CompanyCode = '0001'
PurchasingOrganization = '0001' ) )
CREATE BY \_Item SET FIELDS WITH VALUE #( ( %cid_ref = 'doc1'
%target = VALUE #( ( %cid = 'item1'
itemdescription = 'EML Test Item 1' ) ) ) )
ENTITY Item
CREATE BY \_Account SET FIELDS WITH VALUE #( ( %cid_ref = 'item1'
%target = VALUE #( ( %cid = 'acc1'
costcenter = 'SVC_001' ) ) ) )
MAPPED DATA(mapped)
FAILED DATA(failed)
REPORTED DATA(reported).
COMMIT ENTITIES.
early numbering
in BDEF for each entity where you need to generate and assign primary keysFOR NUMBERING
for each such entitymapped
parameterYou must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
55 | |
5 | |
5 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 | |
3 |