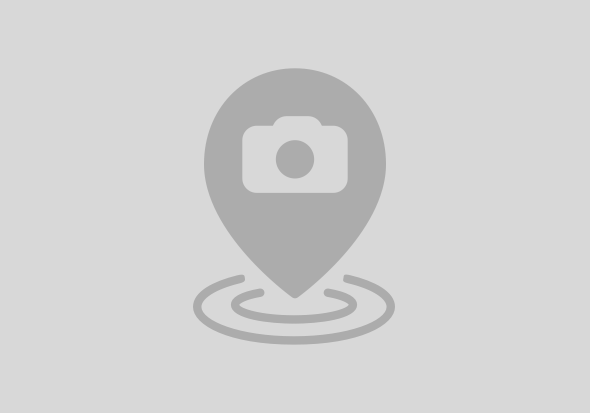
import com.sap.gateway.ip.core.customdev.util.Message;
import java.util.HashMap;
def Message processData(Message message)
{
def body = message.getBody(java.lang.String) as String;
def messageLog = messageLogFactory.getMessageLog(message);
if(messageLog != null)
{
messageLog.setStringProperty("log1","Printing Payload As Attachment")
messageLog.addAttachmentAsString("log1",body,"text/plain");
}
return message;
}
import com.sap.gateway.ip.core.customdev.util.Message;
import java.util.HashMap;
def Message processData(Message message)
{
def map = message.getProperties();
def ex = map.get("CamelExceptionCaught");
if (ex != null)
{
exceptionText = ex.getMessage();
def messageLog = messageLogFactory.getMessageLog(message);
messageLog.addAttachmentAsString("Exception", exceptionText,"application/text");
}
return message;
}
import com.sap.gateway.ip.core.customdev.util.Message;
import java.util.HashMap;
def Message processData(Message message)
{
def body = message.getBody();
sleep(40000);
message.setBody(body);
return message;
}
import com.sap.gateway.ip.core.customdev.util.Message;
import java.util.HashMap;
def Message processData(Message message)
{
def removal=message.getBody(java.lang.String) as String;
removal=removal.replace(/<?xml version="1.0" encoding="UTF-8"?>/,"");
message.setBody(removal);
return message;
}
import com.sap.gateway.ip.core.customdev.util.Message;
import java.util.HashMap;
import java.lang.*;
def Message processData(Message message)
{
map = message.getProperties();
return message;
}
Result:
import com.sap.gateway.ip.core.customdev.util.Message;
import java.util.HashMap;
def Message processData(Message message) {
map = message.getProperties();
def zID = map.get("ID");
def indicator;
if(zID == '')
{
indicator = 'false';
}
else
{
indicator = 'true';
}
message.setProperty("Result", indicator);
return message;
}
Result:
import com.sap.gateway.ip.core.customdev.util.Message;
import java.util.HashMap;
import java.lang.*;
def Message processData(Message message)
{
map = message.getProperties();
def ZF1 = map.get("F1");
def ZF2 = map.get("F2");
def ZF3 = map.get("F3");
String ZConcat = ZF1.concat(ZF2).concat(ZF3);
message.setProperty("ConcatenatedResult",ZConcat);
return message;
}
Result:
import com.sap.gateway.ip.core.customdev.util.Message;
import java.util.HashMap;
import java.lang.*;
import com.sap.it.api.mapping.*;
def Message processData(Message message)
{
Date zcurDate = new Date();
Date zNewDate = zcurDate + 30;
message.setProperty("Current_Date",zcurDate);
message.setProperty("New_Date",zNewDate);
return message;
}
import com.sap.gateway.ip.core.customdev.util.Message;
import java.util.HashMap;
import java.lang.*;
import com.sap.it.api.mapping.*;
def Message processData(Message message)
{
def zNewDate = new Date().plus(30)
message.setProperty("New_Date",zNewDate);
return message;
}
import com.sap.gateway.ip.core.customdev.util.Message;
import java.util.HashMap;
import com.sap.it.api.mapping.*;
def String datePlus30(String header,MappingContext context){
Date zcurDate = new Date();
Date znewDate = zcurDate + 30;
return znewDate.format("yyyyMMdd");
}
import com.sap.it.api.mapping.*;
def String customFunc(String arg1){
return null
}
import com.sap.gateway.ip.core.customdev.util.Message;
import java.util.HashMap;
import com.sap.it.api.mapping.*;
def String getMessageProcessingLogID(String header,MappingContext context)
{
String mplId = context.getHeader("SAP_MessageProcessingLogID").toString();
return mplId;
}
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
11 | |
5 | |
5 | |
5 | |
5 | |
4 | |
4 | |
3 | |
3 | |
3 |