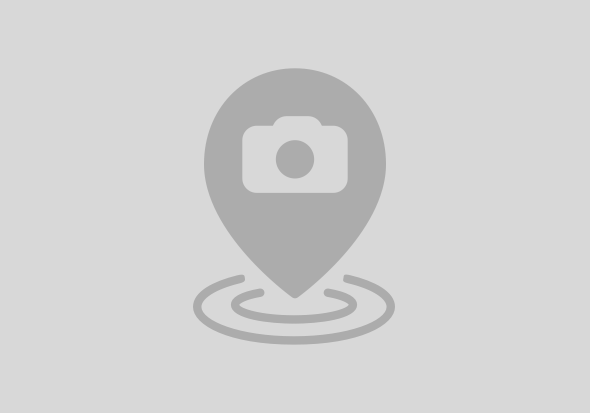
- test
- arrangements
- Common.js
- integration
- pages
- app
- App.js
- ...
- ...
- App.js
- ...
- sinon
- routes
- App.js
- ...
- Utils.js
- sinonServer.js
- AllOPATests.js
- configureAndRequire.js
- index.html
- webapp
- controller
- i18n
- view
- index.html
- Component.js
oServer.respondWith("HEAD", /\/api\/v1\/(?:.+)\/(?:.+)/, [200, {
"Content-Type": "application/json"
}, ""]);
oServer.respondWith("GET", "/api/v1/users/1", [200, {
"Content-Type": "application/json"
}, JSON.stringify([{
"userName": "Jon Doe",
"email": "jon@doe.tld"
}])]);
QUnit.module("Module Name", oModuleConfig);
opaTest("My Atomic test", function(Given, When, Then) {
// Arrangements
Given.iStartMyApp("Users");
Given.componentLoaded();
Given.applicationDataLoaded();
// Actions
When.fillInMyPageObjectName.doSomeAction("Parameter1", "Parameter2");
// Assertions
Then.pageObjectNameUnderTest.assertionFunctionOne("Parameter1");
Then.pageObjectNameUnderTest.assertionFunctionTwo();
});
{
matchers: new sap.ui.test.matchers.PropertyStrictEquals({
name: "text",
value: "To be found value"
}),
}
matchers: function() {
// Workaround with remove to increase speed of testing
var oMessageToast = jQuery(".sapMMessageToast");
var sText = oMessageToast.text();
if (oMessageToast) {
oMessageToast.remove();
}
return sText;
}
/**
* Test if an element has any text and visibility is true.
* @param {string} sViewName - ID of the view.
* @param {string} sId - ID of the to be checked element.
* @param {string} sSuccessText - Success message.
* @returns {object}
*/
iShouldSeeText: function(sViewName, sId, sSuccessText) {
return this.waitFor({
id: sId,
viewName: sViewName,
/**
* Executed when the object is found, visible and animated.
* @param {object} oElement - Found object.
* @returns {void}
*/
success: function(oElement) {
ok((oElement.getText().length > 0), sSuccessText);
},
errorMessage: "Could not find text at view " + sViewName + " and id " + sId
});
},
sap.ui.define([
"sap/ui/test/Opa5",
"test/integration/pages/CommonFunctions"
], function(Opa5, CommonFunctions) {
"use strict";
Opa5.createPageObjects({
onTheApp: {
baseClass: CommonFunctions,
actions: {
},
assertions: new Opa5({
})
}
});
});
/* global checkStart */
jQuery.sap.require("test.configureAndRequire");
// The first require block loads the definitions like shared classes, assertions and so on
sap.ui.require([
"sap/ui/test/Opa5",
"test/arrangements/Common",
"test/integration/pages/app/App",
"test/sinon/routes/App"
], function(Opa5, Common) {
"use strict";
// Define the context of the to be tested application including the base view name
Opa5.extendConfig({
viewNamespace: "your.app.view.",
viewName: "App",
autowait: true,
arrangements: new Common()
});
// Require the individual tests and mocked routes
sap.ui.require([
"test/integration/App"
], function() {
// Only when all files are required, start the Qunit tests.
checkStart();
});
});
/* global QUnit, jQuery, sinon */
var oModuleConfig;// eslint-disable-line no-unused-vars
// Require the testing framework
jQuery.sap.require("sap.ui.qunit.qunit");
jQuery.sap.require("sap.ui.qunit.qunit-css");
jQuery.sap.require("sap.ui.qunit.qunit-junit");
jQuery.sap.require("sap.ui.thirdparty.qunit");
//KarmaJS might have loaded SinonJS
if (typeof sinon === "undefined") {
jQuery.sap.require("sap.ui.thirdparty.sinon");
jQuery.sap.require("sap.ui.thirdparty.sinon-server");
}
jQuery.sap.require("test.sinon.sinonServer");
/**
* Delayed start of QUnit or KarmaJS might break.
* @returns {void}
*/
function checkStart() {// eslint-disable-line no-unused-vars
"use strict";
var aModules, i, iLength;
if (!window["sap-ui-config"] || !window["sap-ui-config"].libs || !sap) {
setTimeout(checkStart, 500);
return;
}
aModules = window["sap-ui-config"].libs.replace(/sap./g, "").replace(/\s/g, "").split(",");
for (i = 0, iLength = aModules.length; i < iLength; i++) {
if ((aModules[i].indexOf(".") !== -1 && !sap[aModules[i].split(".")[0]]) || (aModules[i].indexOf(".") === -1 && !sap[aModules[i]])) {
setTimeout(checkStart, 500);
return;
}
}
QUnit.load();
QUnit.start();
}
/*
* This object will be used whenever a module is defined.
*/
oModuleConfig = {
/**
* Executed before each test.
* @returns {void}
*/
beforeEach: function() {
"use strict";
},
/**
* Executed after each test.
* @returns {void}
*/
afterEach: function() {// eslint-disable-line require-jsdoc/require-jsdoc
"use strict";
// Hacks to ensure the component is teared down even in case an element could not be found
var clock = sinon.useFakeTimers();
new sap.ui.test.Opa5().iTeardownMyUIComponent();
sap.ui.test.Opa.emptyQueue();
clock.tick(1000);
clock.restore();
}
};
// Do not auto start, to ensure all components are loaded
QUnit.config.autostart = false;
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>OPA5 Tests</title>
<script
id="sap-ui-bootstrap"
src="/resources/sap-ui-core.js"
data-sap-ui-libs="sap.m"
data-sap-ui-theme="sap_belize"
data-sap-ui-language="en"
data-sap-ui-compatVersion="edge"
data-sap-ui-preload="async"
data-sap-ui-frameOptions="deny"
data-sap-ui-animation="false"
data-sap-ui-resourceroots='{ "test": "/test", "your.app": "/webapp/" }'>
</script>
</head>
<body>
<div id="qunit-wrapper">
<div id="qunit"></div>
<div id="qunit-fixture"></div>
</div>
<script type="text/javascript">jQuery.sap.require("test.AllOPATests");</script>
</body>
</html>
/* global ok */
sap.ui.define([
"sap/ui/core/routing/HashChanger",
"sap/ui/test/Opa5",
"sap/ui/test/matchers/PropertyStrictEquals"
], function(HashChanger, Opa5, PropertyStrictEquals) {
"use strict";
var Common = Opa5.extend("test.arrangements.Common", {
/**
* Start the app via Component.js for best performance and easier debugging.
* @param {string} sFunctionHash - Manipulate URL to this function name before starting the component.
* @returns {object}
*/
iStartMyApp: function(sFunctionHash) {
var sNewHash = String(sFunctionHash || "");
if (jQuery(".sapUiOpaComponent").length !== 0) {
this.iTeardownMyUIComponent();
}
HashChanger.getInstance().replaceHash(sNewHash);
return this.iStartMyUIComponent({
componentConfig: {
name: "sap.hcp.analytics.mco"
},
hash: sNewHash
});
},
/**
* Arrangement if the component could be loaded.
* @returns {object}
*/
componentLoaded: function() {
return this.waitFor({
viewName: "App",
/**
* Check if jQuery can find a div with the message toast class.
* @returns {boolean}
*/
check: function() {
return (jQuery(".sapUiOpaComponent").length !== 0);
},
/**
* Success callback. Element might be hidden.
* @returns {void}
*/
success: function() {
ok(true, "The component was loaded");
},
/**
* Error callback.
* @returns {void}
*/
error: function() {},
errorMessage: "Could not load component"
});
}
});
return Common;
});
/* global QUnit, oModuleConfig */
sap.ui.require([
"sap/ui/test/Opa5",
"sap/ui/test/opaQunit"
], function(Opa5, opaTest) {
"use strict";
QUnit.module("test/integration/App.js", oModuleConfig);
opaTest("Menu has expected items", function(Given, When, Then) {
// Arrangements
Given.iStartMyApp("404");
Given.componentLoaded();
// Assertions
Then.onTheApp.theMenuHasItems().iTeardownMyUIComponent();
});
});
/* global ok, strictEqual */
sap.ui.define([
"sap/ui/test/Opa5",
"sap/ui/test/matchers/Properties",
"sap/ui/test/matchers/PropertyStrictEquals",
"sap/ui/test/actions/Press"
], function(Opa5, Properties, PropertyStrictEquals, Press) {
"use strict";
var CommonFunctions = Opa5.extend("test.integration.pages.CommonFunctions", {
/**
* Test if an element has any text.
* @param {string} sViewName - ID of the view.
* @param {string} sId - ID of the to be checked element.
* @param {string} sSuccessText - Success message.
* @returns {object}
*/
iShouldSeeSomeText: function(sViewName, sId, sSuccessText) {
return this.waitFor({
id: sId,
viewName: sViewName,
/**
* Executed when the object is found, visible and animated.
* @param {object} oElement - Found object.
* @returns {void}
*/
actions: function(oElement) {
ok(oElement.getText(), sSuccessText);
},
errorMessage: "Could not find any text at view " + sViewName + " and id " + sId
});
}
});
return CommonFunctions;
});
/* global strictEqual, ok */
sap.ui.define([
"sap/ui/test/Opa5",
"test/integration/pages/CommonFunctions"
], function(Opa5, CommonFunctions) {
"use strict";
Opa5.createPageObjects({
onTheApp: {
baseClass: CommonFunctions,
actions: {
},
assertions: new Opa5({
/**
* Check if the site menu has a given list of items.
* @param {string} sViewName - Name of the to be loaded.
* @returns {object}
*/
theMenuHasItems: function(sViewName) {
return this.waitFor({
id: "sideNavigationList",
viewName: "App",
/**
* Executed when the success check becomes true.
* @param {object} oElement - Found object.
* @returns {void}
*/
actions: function(oElement) {
var aItems = oElement.getItems();
strictEqual(jQuery.sap.equal([{
"title": "Home",
"icon": "sap-icon://home",
"key": "Home"
}], [{
"title": aItems[0].getText(),
"icon": aItems[0].getIcon(),
"key": aItems[0].getKey()
}]), true, "The menu contained expected items");
},
errorMessage: "The menu did not contain expected items"
});
}
})
}
});
});
/* global sinon */
// Start the sinon.js fake server
var oServer = sinon.fakeServer.create();
oServer.autoRespond = true;
// Only those requests, which are directed to the SWA models, should be mocked.
oServer.xhr.useFilters = true;
oServer.xhr.addFilter(function(sMethod, sUrl) {
"use strict";
// whenever the regex returns true the request will not faked
return (!sUrl.match(/\/api\/v1\//));
});
/* global oServer */
sap.ui.require([], function() {
"use strict";
oServer.respondWith("HEAD", /\/api\/v1\/(?:.+)\/(?:.+)/, [200, {
"Content-Type": "application/json"
}, ""]);
});
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
13 | |
10 | |
7 | |
6 | |
6 | |
6 | |
6 | |
6 | |
6 | |
6 |