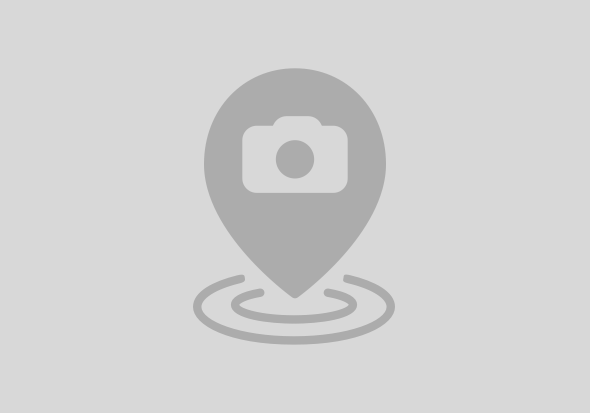
20201231 11:56:35
var strDateTime = "20201231 11:56:35";
var intSpacePlace = strDateTime.indexOf(" ");
console.log("position of space in date/time string: " + intSpacePlace.toString());
Note: Notice the toString method that we are using at the end of our intSpacePlace variable. We need this method to convert the number to text, so that we can concatenate the variable value to the label string.
position of space in date/time string: 8
Note: To access the console, first run the Analytic Application. Then, in Chrome press the F12 key, which will open a code specific area in the browser. Then, click on the Console menu item. You will see some output that we did not send, but at the bottom you should see our output.
strDateTime.subString(intSpacePlace)
Code: console.log("time with leading space: " + strDateTime.substring(intSpacePlace));
console output: time with leading space: 11:56:35
Code:
intSpacePlace = intSpacePlace + 1;
console.log("time with no leading space: " + strDateTime.substring(intSpacePlace));
Output:
time with no leading space: 11:56:35
Code:
var strTimeWithSpace = strDateTime.substring(strDateTime.indexOf(" "));
console.log("time with leading space trimmed: " + strTimeWithSpace.trim());
Output:
time with no leading space: 11:56:35
// Date string with space //
// Want to extract time
//--------------------------------------------------------------------------//
console.log("// Want to extract time");
console.log("// 20201231 11:56:35");
var strDateTime = "20201231 11:56:35";
// Three step solution -------------------------------------
// Locate the space
var intSpacePlace = strDateTime.indexOf(" ");
console.log("position of space in date/time string: " + intSpacePlace.toString());
// Extract time, but with leading space
console.log("time with leading space: " + strDateTime.substring(intSpacePlace));
// Eliminate space by adding one to space position
intSpacePlace = intSpacePlace + 1;
// Use subString to retrieve time with no space
console.log("time with no leading space: " + strDateTime.substring(intSpacePlace));
// Could do it in one line----------------------------------
console.log("time with no leading space: " + strDateTime.substring(strDateTime.indexOf(" ")+1));
// Instead of adding 1 to get to space after ---------------
// Could use Trim instead
var strTimeWithSpace = strDateTime.substring(strDateTime.indexOf(" "));
console.log("time with leading space trimmed: " + strTimeWithSpace.trim());
console.log("");
//--------------------------------------------------------------------------//
var strEmbedded = "The red ball";
Code: var intColorPos = strEmbedded.indexOf(" ");
console.log("Space before color position: " + intColorPos.toString());
Output: Space before color position: 3
Code: var intColorEnd = strEmbedded.lastIndexOf(" ");
console.log("Space after color position: " + intColorEnd.toString());
Output: Space after color position: 7
Code:
var strColorWithSpace = strEmbedded.substr(intColorPos, intColorEnd-intColorPos);
console.log("The color is (no space management): " + strColorWithSpace);
var strNoSpace = strEmbedded.substr(intColorPos, intColorEnd-intColorPos).trim();
console.log("The color is (with space management): " + strNoSpace );
Output:
The color is (no space management): red
The color is (with space management): red
// subStr uses start and length
// intColorPos is start
// intColorEnd-intColorPos is length
console.log("subStr: " + strEmbedded.substr(intColorPos,intColorEnd-intColorPos));
// subString uses start and end
// intColorPos is start
// intColorEnd is end
console.log("subString: " + strEmbedded.substring(intColorPos, intColorEnd));
// Embedded text //
//--------------------------------------------------------------------------//
console.log("// Want to extract embedded color");
console.log("// The red ball");
var strEmbedded = "The red ball";
// Want to extract the color of the ball
// Find position of color
var intColorPos = strEmbedded.indexOf(" ");
console.log("Space before color position: " + intColorPos.toString());
// Find Length of color
var intColorEnd = strEmbedded.lastIndexOf(" ");
console.log("Space after color position: " + intColorEnd.toString());
// isolate color with spaces
var strColorWithSpace = strEmbedded.substr(intColorPos, intColorEnd-intColorPos);
console.log("The color is (no space management): " + strColorWithSpace);
// isolate color with no spaces
var strNoSpace = strEmbedded.substr(intColorPos, intColorEnd-intColorPos).trim();
console.log("The color is (with space management): " + strNoSpace );
// subStr uses start and length
// intColorPos is start
// intColorEnd-intColorPos is length
console.log("subStr: " + strEmbedded.substr(intColorPos,intColorEnd-intColorPos));
// subString uses start and end
// intColorPos is start
// intColorEnd is end
console.log("subString: " + strEmbedded.substring(intColorPos, intColorEnd));
console.log("");
var strDescLine = "Diameter: 15 cm; Color: blue; Weight: 15 kg";
var strDelimiter = "color: ";
var intDelimiterLength = strDelimiter.length;
Code:
var intDelimiterPos = strDelimiter.indexOf(strDelimiter);
console.log("Doesn't find delimiter (different case): " + intDelimiterPos.toString());
Output:
Doesn't find delimiter (different case): 0
Code: var strDescLineLC = strDescLine.toLowerCase();
console.log("lower case string: " + strDescLineLC);
Output: lower case string: diameter: 15 cm; color: blue; weight: 15 kg
Code: intDelimiterPos = strDescLineLC.indexOf(strDelimiter);
console.log("delimiter position: " + intDelimiterPos.toString());
Output: delimiter position: 17
Code:
var strStartWithColor = strDescLineLC.substring(intDelimiterPos+intDelimiterLength);
console.log("string starting with color: " + strStartWithColor);
Output:
string starting with color: blue; weight: 15 kg
Code:
var strEmbeddedColor = strStartWithColor.substring(0, strStartWithColor.indexOf(";"));
console.log("color is: " + strEmbeddedColor );
Output:
color is: blue
Code:
var strOneLiner = strDescLineLC.substring(strDescLineLC.indexOf(strDelimiter)+strDelimiter.length, strDescLineLC.indexOf(";", strDescLineLC.indexOf(strDelimiter)+strDelimiter.length));
Output:
One line of code: blue
Note: Many coders will break code down into several lines or assignments, so that the code is easier to read, maintain and modify.
// Deeply embedded text //
//--------------------------------------------------------------------------//
console.log("// Want to extract deeply embedded color");
console.log("// Diameter: 15 cm; Color: blue; Weight: 15 kg");
var strDescLine = "Diameter: 15 cm; Color: blue; Weight: 15 kg";
// Want to extract the color of the ball
// Find position of color
var strDelimiter = "color: ";
var intDelimiterLength = strDelimiter.length;
var intDelimiterPos = strDelimiter.indexOf(strDelimiter);
// Doesn't find dimimiter, because color: <> Color:
console.log("Doesn't find delimiter (different case): " + intDelimiterPos.toString());
// Convert to common case
var strDescLineLC = strDescLine.toLowerCase();
console.log("lower case string: " + strDescLineLC);
// Find position of color
intDelimiterPos = strDescLineLC.indexOf(strDelimiter);
// finds dimimiter, because color: = color:
console.log("delimiter position: " + intDelimiterPos.toString());
// Extract string starting with color
var strStartWithColor = strDescLineLC.substring(intDelimiterPos+intDelimiterLength);
console.log("string starting with color: " + strStartWithColor);
// Extract color
var strEmbeddedColor = strStartWithColor.substring(0, strStartWithColor.indexOf(";"));
console.log("color is: " + strEmbeddedColor );
// One line solution
var strOneLiner = strDescLineLC.substring(strDescLineLC.indexOf(strDelimiter)+strDelimiter.length, strDescLineLC.indexOf(";", strDescLineLC.indexOf(strDelimiter)+strDelimiter.length));
console.log("One line of code: " + strOneLiner);
Code: var intNumbersToPad = ["1", "12", "123", "1234"];
var strX = "X";
for(var i = 0; i<4; i++){
console.log(intNumbersToPad[i]);}
Output: 1
12
123
1234
Code:
for (i=0; i<4; i++){
console.log(strX.repeat(4-intNumbersToPad[i].length) + intNumbersToPad[i]);}
Output: XXX1
XX12
X123
1234
// Pad with X's //
//--------------------------------------------------------------------------//
var intNumbersToPad = ["1", "12", "123", "1234"];
var strX = "X";
console.log("// Want to pad to four places using X");
for(var i = 0; i<4; i++){
console.log(intNumbersToPad[i]);}
// Padded output
for (i=0; i<4; i++){
console.log(strX.repeat(4-intNumbersToPad[i].length) + intNumbersToPad[i]);}
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
10 | |
9 | |
8 | |
7 | |
7 | |
6 | |
6 | |
5 | |
5 | |
5 |