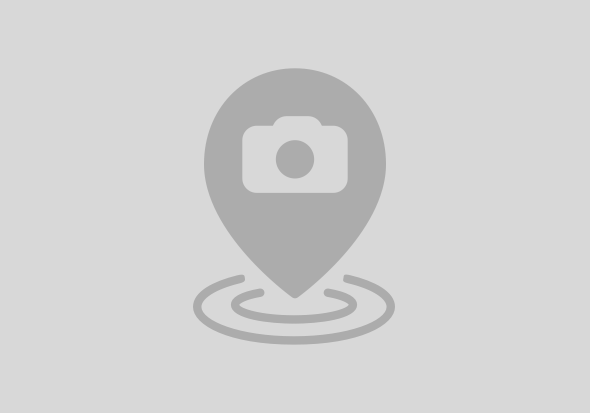
Hi All,
This document defines the procedure of transferring data from c#.net programming to sap backend database tables. It needs to install two components on the client PC on which want to transfer data from .NET to SAP.
1. Dot NET framework 4.0
2. SAP Dot NET connector 3.0 - SAP .NET Connector 3.0 is the current version of SAP's development environment for communication between the Microsoft .NET platform and SAP systems. This connector supports RFCs and Web services. It allows you to write different applications such as Web form, Windows form, or console applications in the Microsoft Visual Studio.Net. With the SAP .NET Connector, you can use all common programming languages, such as Visual Basic. NET, C#, or Managed C++. Write .NET Windows and Web form applications that have access to SAP business objects (BAPIs).
.NET connector 3.0 is available on SAP market place.
Procedure to make BAPI and object type on SAP side:
1. First make a structure for bapi, structure name should start with Z OR Y following by BAPI . e.x. YBAPI_ATND_RAW
2. Now make a module function which is RFC enabled. So, go to SE37 tcode and give the name as Z OR Y following by BAPI like ZBAPI_ATTENDANCE_PUNCH_DATA and in attributes tick on Remote-Enabled Module and tick on Start immed as shown below:
3. Must define the export parameter return type bapireturn.
4. Define the parameter name in tables as shown below:
5. Write the source code:
*"----------------------------------------------------------------------
*"*"Local Interface:
*" EXPORTING
*" VALUE(RETURN) TYPE BAPIRETURN
*" TABLES
*" ITAB STRUCTURE YBAPI_ATND_RAW
*"----------------------------------------------------------------------
TABLES : yatnrcraw.
DATA : v_srno(5) TYPE n,
wa_itab3 LIKE LINE OF itab.
DATA : BEGIN OF itab4 OCCURS 0.
INCLUDE STRUCTURE yatnrcraw.
DATA : END OF itab4.
CLEAR v_srno.
LOOP AT itab INTO wa_itab3.
v_srno = v_srno + 1.
CONDENSE wa_itab3-tmp.
* CONCATENATE wa_itab3-tmp+24(2) '.' wa_itab3-tmp+22(2) '.' wa_itab3-tmp+18(4) INTO yatndent-pchdt.
yatnrcraw-ardata = wa_itab3-tmp.
CONCATENATE wa_itab3-tmp+18(4)
wa_itab3-tmp+22(2)
wa_itab3-tmp+24(2) INTO yatnrcraw-pchdt.
CONCATENATE wa_itab3-tmp+26(2)
wa_itab3-tmp+28(2) '00' INTO yatnrcraw-time.
yatnrcraw-mcno = wa_itab3-tmp+31(7).
yatnrcraw-empcd = wa_itab3-tmp+12(6).
WRITE wa_itab3-tmp+30(1) TO yatnrcraw-ioind.
CALL FUNCTION 'CONVERSION_EXIT_ALPHA_INPUT'
EXPORTING
input = yatnrcraw-empcd
IMPORTING
output = yatnrcraw-empcd.
yatnrcraw-upddt = sy-datum.
yatnrcraw-uname = sy-uname.
** INSERT yatnrcraw FROM yatnrcraw.
INSERT yatnrcraw INTO TABLE itab4.
CLEAR yatnrcraw.
ENDLOOP.
IF itab4[] IS NOT INITIAL.
SORT itab4 BY pchdt empcd time.
DELETE ADJACENT DUPLICATES FROM itab4 COMPARING pchdt empcd time.
LOOP AT itab4.
SELECT *
FROM yatnrcraw
WHERE ardata = itab4-ardata.
ENDSELECT.
IF sy-subrc EQ 0.
CLEAR itab4.
CONTINUE.
ENDIF.
INSERT yatnrcraw FROM itab4.
CLEAR itab4.
ENDLOOP.
ENDIF.
* IF v_srno GT 0.
* MESSAGE 'Data has successfully uploaded' TYPE 'I'.
* ENDIF.
Activate the module.
NOTE: above code is just an example, define the tables, import and export parameters as per your requirement and develop the logic as per your requirement. Must define the RETURN parameter in export.
6. Create the API Method Using the BAPI WIZARD: Develop the object type using the Business Object builder tcode SWO1. Ex. Give the name ZATND
7 . The next step is to add the ZBAPI_ATTENDANCE_PUNCH_DATA method to the business object. Select Utilities -> API methods -> Add method and write the name of the function module in the dialog box. Next the dialog ox show below will be shown. This is the start screen of the BAPI wizard
Then click on OK then get the below screen.
Then click on next button get the below screen, which defines all the import, export parameters. Here in method parameter and name comes by default as same as name in function module. If requires change teh parameter name in object type.
Get a screen and click on OK.
After finished the wizard, notice that the ZbapiPunchData method has been added to the business object.
Double-click on the method to see its properties. To use the business object you must change the Object type status to Implemented. Use menu Edit->Change releases status->Object type->To implemented. No you can test the object (Press F8).
Note that the BAPI wizard has added a wrapper class for the function module so it can be ued as method in the business object. Choose menu Goto->Program to display the program:
***** Implementation of object type ZATND1 *****
INCLUDE <OBJECT>.
BEGIN_DATA OBJECT. " Do not change.. DATA is generated
* only private members may be inserted into structure private
DATA:
" begin of private,
" to declare private attributes remove comments and
" insert private attributes here ...
" end of private,
KEY LIKE SWOTOBJID-OBJKEY.
END_DATA OBJECT. " Do not change.. DATA is generated
BEGIN_METHOD ZBAPIPUNCHDATA CHANGING CONTAINER.
DATA:
RETURN LIKE BAPIRETURN,
ITAB LIKE YBAPI_ATND_RAW OCCURS 0.
SWC_GET_TABLE CONTAINER 'Itab' ITAB.
CALL FUNCTION 'ZBAPI_ATTENDANCE_PUNCH_DATA'
IMPORTING
RETURN = RETURN
TABLES
ITAB = ITAB
EXCEPTIONS
OTHERS = 01.
CASE SY-SUBRC.
WHEN 0. " OK
WHEN OTHERS. " to be implemented
ENDCASE.
SWC_SET_ELEMENT CONTAINER 'Return' RETURN.
SWC_SET_TABLE CONTAINER 'Itab' ITAB.
END_METHOD.
Now click on this to generate the object and get back to initial screen. Follow the path Object Type -> Change Release Status to -> Released.
Release the BAPI as a method in the BOR (Release the methods you has created - Set the cursor on the method then
Edit -> Change release status -> Object type component -> To released)
Procedure to develop code in Microsoft Visual C#. NET:
1. Develop a new project named as DataToSap and go to the form open the code
2. Open the solution manager and right click on References and add reference. Go to the BROWSE tab and add the components from the location as path shown below:
C:\Program Files\SAP\SAP_DotNetConnector3_x86
And the components as shown below:
Click on OK, now both the components are added.
3. Add the namespance using SAP.Middleware.Connector;
And copy the following code...
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
SetupUI();
}
private void SetupUI()
{
try
{
WriteToFile();
}
catch (Exception)
{
System.Environment.Exit(0);
}
}
private void WriteToFile()
{
try
{
RfcConfigParameters rfc = new RfcConfigParameters();
rfc.Add(RfcConfigParameters.Name, "mySapName");
rfc.Add(RfcConfigParameters.AppServerHost, "10.147.1.3");
rfc.Add(RfcConfigParameters.Client, "410");
rfc.Add(RfcConfigParameters.User, "JSTIT");
rfc.Add(RfcConfigParameters.Password, "unitech@1");
rfc.Add(RfcConfigParameters.SystemNumber, "00");
rfc.Add(RfcConfigParameters.Language, "EN");
rfc.Add(RfcConfigParameters.PoolSize, "5");
rfc.Add(RfcConfigParameters.PeakConnectionsLimit, "10");
rfc.Add(RfcConfigParameters.ConnectionIdleTimeout, "500");
RfcDestination rfcDest = RfcDestinationManager.GetDestination(rfc);
RfcRepository rfcRep = rfcDest.Repository;
IRfcFunction function = rfcRep.CreateFunction("ZBAPI_ATTENDANCE_PUNCH_DATA");
IRfcTable table = function.GetTable("Itab");
table.Insert();
table.SetValue("TMP", "00001100000010057 20130918933I0000101");
function.Invoke(rfcDest);
table.Insert();
table.SetValue("TMP", "00001100000010057 20130919933I0000101");
function.Invoke(rfcDest);
}
catch (Exception e)
{
MessageBox.Show(e.Message.ToString());
}
}
}
4. Also copy all the dll files of .net connector folder which is in SAP folder in program files of C drive and paste in the bin folder of the .net project.
NOTE: 1. above program is just an example for getting only knowledge on this.
2. Also need some knowledge on C#.NET.
Many Thanks / Himanshu Gupta
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
4 | |
3 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 | |
1 |