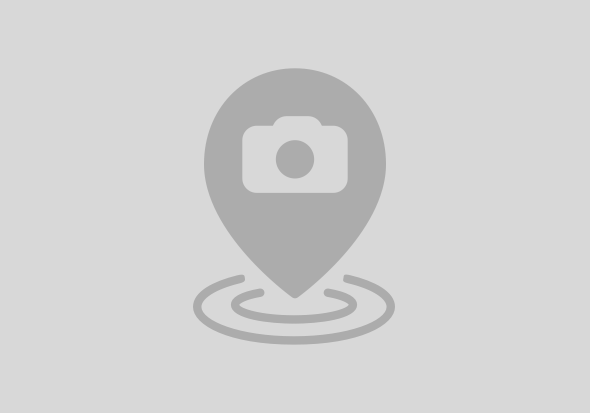
"@sap-cloud-sdk/http-client": "^3.9.0",
const httpclient = require('@sap-cloud-sdk/http-client');
await httpclient.executeHttpRequest(
{
destinationName: 'MYDESTINATION'
},
{
method: 'POST',
url: "/sap/opu/odata/sap/ZMY_SERV_SRV/MyEntitySet",
headers: {
"Content-Type":"application/json; charset=utf-8",
"Accept":"application/json"
},
data: body
}
).then(response => {
// use eg response.status and response.data
}).catch(err => {
// use eg err.code or message
});
...
modules:
- name: myproxy
type: nodejs
requires:
- name: myproxy-destination-service
parameters:
content-target: true
- name: myproxy-connectivity-service
parameters:
health-check-type: process
...
resources:
- name: myproxy-destination-service
type: org.cloudfoundry.managed-service
parameters:
config:
version: 1.0.0
service: destination
service-name: myproxy-destination-service
service-plan: lite
- name: myproxy-connectivity-service
type: org.cloudfoundry.managed-service
parameters:
service: connectivity
service-plan: lite
"express": "^4.17.3",
"body-parser": "^1.20.2",
"passport": "^0.6.0",
"passport-http": "^0.3.0"
const express = require('express');
const bodyParser = require('body-parser')
const passport = require('passport');
const passportHTTP = require('passport-http');
const auth_env = {login: process.env['AUTH_LOGIN'], password: process.env['AUTH_PASSWORD']};
const app = express();
passport.use(new passportHTTP.BasicStrategy(
function(username, password, done) {
if (username === auth_env.login && password === auth_env.password) {
return done(null, username);
} else {
return done(null, false);
}
}
));
app.use(passport.initialize());
app.use(passport.authenticate('basic', { session: false }));
app.use(bodyParser.text({ type: 'application/json' }));
...
app.listen(process.env.PORT || 5000, function () {
console.log('Proxy app started');
});
app.post('/MyEntitySet', async (req, res) => {
if (!req.user) { res.sendStatus(403); return; }
await httpclient.executeHttpRequest(
{
destinationName: 'MY_DESTINATION'
},
{
method: 'POST',
url: "/sap/opu/odata/sap/ZMY_SERV_SRV/MyEntitySet",
headers: {
"Content-Type":"application/json; charset=utf-8",
"Accept":"application/json"
},
data: req.body
}
).then(response => {
res.send(response.data);
}).catch(err => {
res.status(500).send('Backend Error');
});
});
{
"scopes": [
{
"name": "$XSAPPNAME.access",
"description": "Access"
}
],
"role-templates": [
{
"name": "Access",
"default-role-name": "My Proxy Access Authorization",
"scope-references": [
"$XSAPPNAME.access"
]
}
],
"oauth2-configuration": {
"redirect-uris": [
"http://localhost:9999/success"
]
}
}
requires:
- name: my_proxy-uaa
...
resources:
- name: my_proxy-uaa
type: org.cloudfoundry.managed-service
parameters:
path: ./xs-security.json
service-plan: application
service: xsuaa
config:
xsappname: my_proxy-${space}
tenant-mode: dedicated
role-collections:
- name: 'my_proxy-Access-${space}'
role-template-references:
- $XSAPPNAME.Access
"@sap/xsenv": "^4.2.0",
"@sap/xssec": "^3.6.0",
const httpclient = require('@sap-cloud-sdk/http-client');
const express = require('express');
const bodyParser = require('body-parser')
const xsenv = require('@sap/xsenv');
const passport = require('passport');
const xssec = require('@sap/xssec');
xsenv.loadEnv();
const app = express();
const services = xsenv.getServices({ uaa: 'my_proxy-uaa' });
passport.use(new xssec.JWTStrategy(services.uaa));
app.use(passport.initialize());
app.use(passport.authenticate('JWT', { session: false }));
app.use(bodyParser.text({ type: 'application/json' }));
app.post('/MyEntitySet', async (req, res) => {
if (!req.authInfo.checkLocalScope('access')) {
return res.status(403).send('Forbidden');
}
await httpclient.executeHttpRequest(
{
destinationName: 'MY_DESTINATION'
jwt: req.authInfo.getAppToken()
},
{
method: 'POST',
url: "/sap/opu/odata/sap/ZMY_SERV_SRV/MyEntitySet",
headers: {
"Content-Type":"application/json; charset=utf-8",
"Accept":"application/json"
},
data: req.body
}
)
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
9 | |
8 | |
5 | |
5 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 |