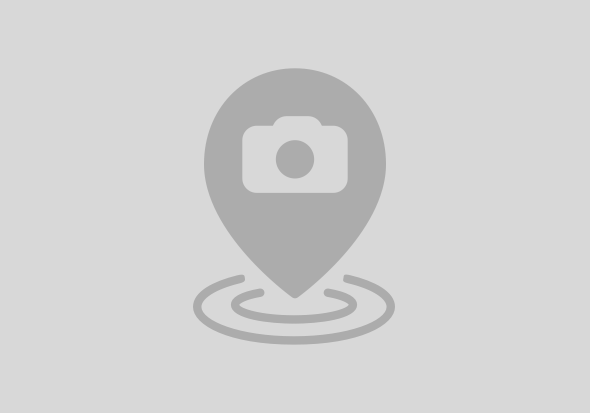
DATA: gv_uri TYPE string,
gv_payload TYPE string,
go_client TYPE REF TO cl_http_client,
ldate TYPE d,
sdate TYPE d,
edate TYPE d,
date_str TYPE string,
sdate_str TYPE string,
edate_str TYPE string,
sum_tabix TYPE i,
str_bonus TYPE string VALUE ' ', " Initialized to 20 spaces
http_client_instance TYPE REF TO any, " Replace 'any' with the actual class type
request_payload TYPE string,
response_data TYPE string,
json_data_root TYPE REF TO /ui2/cl_json,
json_data_node TYPE REF TO any, " Replace 'any' with the appropriate class or data type
lv_seqnr TYPE zuspy_dg_sb_log-seqnr,
field_ref TYPE REF TO data,
val_ref TYPE REF TO data,
lv_abap_date TYPE d,
lv_date_in_milliseconds TYPE n LENGTH 13,
str_date TYPE string,
lv_result_string TYPE string,
lv_seconds_since_epoch TYPE p DECIMALS 0,
c_epoch TYPE d VALUE '19700101',
c_seconds_in_a_day TYPE i VALUE 86400,
c_milliseconds_in_a_second TYPE i VALUE 1000.
DATA: BEGIN OF json_parsed_data,
editstatus TYPE string,
httpcode TYPE string,
index TYPE string,
inlineresults TYPE string,
key TYPE string,
message TYPE string,
status TYPE string,
END OF json_parsed_data.
FIELD-SYMBOLS: <root_data>,
<child_data>,
<node_data>,
<node_element>,
<element_data>.
clear: gv_uri, gv_payload.
call method cl_http_client=>create_by_destination
exporting
destination = 'ECP_PTP_110' "SM59 HTTP Destination for EC
importing
client = go_client
exceptions
argument_not_found = 1
destination_not_found = 2
destination_no_authority = 3
plugin_not_active = 4
internal_error = 5
others = 6.
if sy-subrc <> 0.
exit.
endif.
gv_uri
is the OData API uri assigned the upsert operation. For more detailed information about edit operations, refer to the documentation. gv_uri = '/odata/v2/upsert?workflowConfirmed=false&$format=json'.
loop at it_dg_rptdata_d_summary into wa_dg_rptdata_d_summary where remaining_bonus is not initial.
move-corresponding wa_dg_rptdata_d_summary to wa_zuspy_dg_sb_log.
sum_tabix = sy-tabix.
perform convert_date using p_date changing date_str.
perform convert_date using wa_dg_rptdata_d_summary-inv_begda changing sdate_str.
perform convert_date using wa_dg_rptdata_d_summary-inv_endda changing edate_str.
clear str_bonus.
str_bonus = wa_dg_rptdata_d_summary-remaining_bonus .
concatenate '{"__metadata":{"uri": "EmpPayCompNonRecurring","type": "SFOData.EmpPayCompNonRecurring"},'
'"payDate": "' date_str '",'
'"userId": "' wa_dg_rptdata_d_summary-ecid '",'
'"payComponentCode": "' p_lgart '",'
'"value": "' str_bonus '",'
'"currencyCode": "' 'USD' '",'
'"notes": "' wa_dg_rptdata_d_summary-btrtl '",'
'"nonRecurringPayPeriodStartDate":"' sdate_str '",'
'"nonRecurringPayPeriodEndDate":"' edate_str '" }'
into gv_payload.
http_client_instance->request->set_method( 'POST' ).
http_client_instance->request->set_content_type( 'application/json' ).
http_client_instance->request->set_cdata( request_payload ).
" Sending the request
http_client_instance->send(
EXCEPTIONS
http_communication_failure = 1
http_invalid_state = 2 ).
" Receiving the response
http_client_instance->receive(
EXCEPTIONS
http_communication_failure = 1
http_invalid_state = 2
http_processing_failed = 3 ).
CLEAR response_data.
response_data = http_client_instance->response->get_cdata().
CLEAR json_data_root.
json_data_root = /ui2/cl_json=>generate(json = response_data).
IF json_data_root IS BOUND.
ASSIGN json_data_root->* TO <root_data>.
ASSIGN COMPONENT `D` OF STRUCTURE <root_data> TO <child_data>.
IF <child_data> IS ASSIGNED.
json_data_node = <child_data>.
ASSIGN json_data_node->* TO <node_data>.
LOOP AT <node_data> ASSIGNING <node_element>.
IF <node_element> IS ASSIGNED.
ASSIGN <node_element>->* TO <element_data>.
PERFORM extract_from_json USING 'EDITSTATUS' CHANGING json_parsed_data-editstatus.
PERFORM extract_from_json USING 'HTTPCODE' CHANGING json_parsed_data-httpcode.
PERFORM extract_from_json USING 'INDEX' CHANGING json_parsed_data-index.
PERFORM extract_from_json USING 'INLINERESULTS' CHANGING json_parsed_data-inlineresults.
PERFORM extract_from_json USING 'KEY' CHANGING json_parsed_data-key.
PERFORM extract_from_json USING 'MESSAGE' CHANGING json_parsed_data-message.
PERFORM extract_from_json USING 'STATUS' CHANGING json_parsed_data-status.
ENDIF.
ENDLOOP.
ENDIF.
ENDIF.
if json_parsed_data-status = 'OK'.
wa_dg_rptdata_s-status = 'Success'.
modify it_dg_rptdata_d_summary from wa_dg_rptdata_d_summary index sum_tabix.
else. " refine error handling as per your requirement
wa_dg_rptdata_s-status = 'Bonus updated failed'.
modify it_dg_rptdata_d_summary from wa_dg_rptdata_d_summary index sum_tabix.
endif.
endloop.
*************************************************************************************************
FORM extract_from_json USING field_name TYPE string CHANGING target_value.
DATA: field_ref TYPE REF TO data,
val_ref TYPE REF TO data.
ASSIGN COMPONENT field_name OF STRUCTURE <element_data> TO <field_ref>.
IF <field_ref> IS ASSIGNED.
ASSIGN <field_ref>->* TO <val_ref>.
IF <val_ref> IS ASSIGNED.
target_value = <val_ref>.
UNASSIGN: <field_ref>.
CLEAR: <val_ref>.
ENDIF.
ENDIF.
ENDFORM.
*************************************************************************************************
form convert_date using p_date changing c_date_str.
* Calculate the number of days between the ABAP date and the epoch
lv_seconds_since_epoch = ( p_date - c_epoch ) * c_seconds_in_a_day.
* Convert to milliseconds
lv_date_in_milliseconds = lv_seconds_since_epoch * c_milliseconds_in_a_second.
str_date = lv_date_in_milliseconds.
* Format the result
concatenate '/Date(' lv_date_in_milliseconds ')/' into lv_result_string.
c_date_str = lv_result_string.
endform.
*********************************************************************************************
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
13 | |
3 | |
3 | |
2 | |
2 | |
2 | |
2 | |
2 | |
2 | |
1 |